创建一个类 用链表储存超大整数并进行运算
时间: 2023-08-04 18:26:40 浏览: 53
好的,我可以为您解答。为了实现用链表储存超大整数并进行运算,我们可以创建一个类来表示这个超大整数。以下是一个简单的 C++ 代码示例,用于实现这个类:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 链表节点定义
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 超大整数类定义
class BigInt {
private:
ListNode* head; // 链表头指针
public:
BigInt() : head(NULL) {} // 构造函数,初始化链表头指针为空
BigInt(string str) : head(NULL) { // 构造函数,根据字符串初始化链表
for (int i = str.size() - 1; i >= 0; i--) {
ListNode* node = new ListNode(str[i] - '0');
node->next = head;
head = node;
}
}
// 拷贝构造函数,用于拷贝一个 BigInt 对象
BigInt(const BigInt& other) : head(NULL) {
ListNode* cur = other.head;
while (cur != NULL) {
ListNode* node = new ListNode(cur->val);
node->next = head;
head = node;
cur = cur->next;
}
}
// 析构函数,释放链表内存
~BigInt() {
ListNode* cur = head;
while (cur != NULL) {
ListNode* next = cur->next;
delete cur;
cur = next;
}
}
// 重载加号运算符,实现两个 BigInt 对象的加法运算
BigInt operator+(const BigInt& other) const {
BigInt sum;
ListNode* p = head, * q = other.head;
int carry = 0;
while (p != NULL || q != NULL) {
int x = (p != NULL) ? p->val : 0;
int y = (q != NULL) ? q->val : 0;
int s = x + y + carry;
carry = s / 10;
ListNode* node = new ListNode(s % 10);
node->next = sum.head;
sum.head = node;
if (p != NULL) p = p->next;
if (q != NULL) q = q->next;
}
if (carry > 0) {
ListNode* node = new ListNode(carry);
node->next = sum.head;
sum.head = node;
}
return sum;
}
// 重载输出运算符,用于将 BigInt 对象输出到标准输出流中
friend ostream& operator<<(ostream& os, const BigInt& num) {
ListNode* cur = num.head;
while (cur != NULL) {
os << cur->val;
cur = cur->next;
}
return os;
}
};
int main() {
BigInt a("12345678901234567890");
BigInt b("98765432109876543210");
BigInt c = a + b;
cout << c << endl;
return 0;
}
```
在这个示例中,我们创建了一个名为 `BigInt` 的类,用于表示超大整数。这个类的数据成员是一个链表头指针,每个节点包含一个整数。我们在类中实现了构造函数、拷贝构造函数、析构函数、重载加号运算符和输出运算符。
在 `operator+` 函数中,我们使用了链表的基本操作,遍历两个链表并逐位相加,得到一个新的链表表示结果。在 `operator<<` 函数中,我们使用了输出流操作符 `<<`,将链表中的整数逐位输出到标准输出流中。
最后,在 `main` 函数中,我们创建了两个超大整数,并进行了加法运算,将结果输出到标准输出流中。
相关推荐
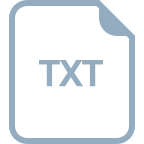
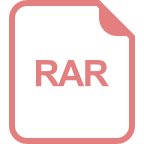
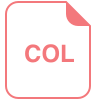
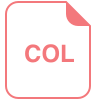
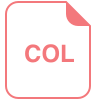











