void ComputePolylist(Polylist L)/*计算多项式在x=%d的值,函数用于计算多项式在某个值上的结果*/ { double x; double sum=0; scanf("%lf",&x); Polylist temp=L->next; while(temp->next!=NULL){ sum=sum+(temp->coef*x); temp=temp->next; } sum=sum+(temp->coef*x); printf("ComputePolylist in x=%.2f result is:%.2f\n",x,sum); } void DestroyPolylist(Polylist L) { Polylist list = L; list=NULL; printf("destroy");为什么这样做
时间: 2024-03-22 18:40:42 浏览: 26
这段代码中的 `ComputePolylist` 函数用于计算多项式在某个值上的结果,而 `DestroyPolylist` 函数用于销毁一个多项式链表。
在 `ComputePolylist` 函数中,首先通过 `scanf` 函数读取一个浮点数 `x`,然后遍历多项式链表,计算每一项系数与 `x` 的乘积,并加到总和 `sum` 中。最后输出计算结果。
在 `DestroyPolylist` 函数中,将传入的多项式链表 `L` 赋值为 `NULL`,相当于将该链表的头节点指针指向了空地址,从而使该链表变为了空链表。同时,输出一条提示信息表明该函数已经执行完毕。
需要注意的是,在 `DestroyPolylist` 函数中,只是将链表的头节点指针赋值为空,并没有释放每个节点占用的内存空间,因此在实际应用中,如果多次创建和销毁多项式链表,可能会导致内存泄漏问题。
相关问题
Polylist AddPolylist()/*创建两个多项式并相加,完成后显示序列*/ { Polylist x,y,a,b,tail,tl,temp; double sum=0; x=CreatePolylist(); y=CreatePolylist(); a=x->next;/*x->next赋给a*/ b=y->next; tail =(Polylist)malloc(sizeof(Polynode)) ; tail->next=NULL; tl=tail; while(a!=NULL&&b!=NULL){ if(a->exp<b->exp){/*a的指数小于b的指数*/ tl->next=a; tl=tl->next; a=a->next; }else if(a->exp=b->exp){/*a的指数等于b的指数*/ sum = a->coef+b->coef; if(sum!=0){ a->coef=sum; tl->next=a; tl=tl->next; a=a->next; b=b->next; }else{ temp =a; a=a->next; free(temp);/*释放temp*/ temp=b; b=b->next; free(temp); } }else{ tl->next=b; tl=tl->next; b=b->next; } } if(a!=NULL){ tl->next=a; }else tl->next=b; return tail; } void ComputePolylist(Polylist L)/*计算多项式在x=%d的值*/ { double x; double sum=0; scanf("%lf",&x); Polylist temp=L->next; while(temp->next!=NULL){ sum=sum+(temp->coef*x); temp=temp->next; } sum=sum+(temp->coef*x); printf("ComputePolylist in x=%.2f result is:%.2f\n",x,sum); } void DestroyPolylist(Polylist L) { Polylist list = L; list=NULL; printf("destroy"); }什么意思
这是一段 C 语言代码,主要是实现多项式的加法、计算多项式在某个值上的结果以及销毁多项式的功能。其中,CreatePolylist() 函数用于创建多项式链表;AddPolylist() 函数用于创建两个多项式并相加;ComputePolylist() 函数用于计算多项式在某个值上的结果;DestroyPolylist() 函数用于销毁多项式链表。具体实现过程可以参考代码注释。
void InitPolylist(Polylist *L) {*L = (Polylist)malloc(sizeof(Polylist)); (*L)->next = NULL; } Polylist CreatePolylist()/*输入多项式的系数和指数,用尾插法建立一元多项式的链表*/ {Polylist L; Polynode *s; InitPolylist(&L); double coef; int exp; Polynode *r = L; scanf("%lf %d",&coef,&exp); while(coef != 0) { s = (Polynode *)malloc(sizeof(Polynode)); s->coef = coef; s->exp = exp; s->next = NULL; r->next = s; r = s; scanf("%lf %d",&coef,&exp); } r->next = NULL; return L; } int LengthPolylist(Polylist L) {Polylist r = L; int n = 0; while(r->next != NULL) { n++; r = r->next; } return n; } void OutputPolylist(Polylist L) { Polylist r = L->next; printf("inlcude %d coef/exp list is:\n",LengthPolylist(L)); while(r != NULL) { printf("%.2f,%d\n",r->coef,r->exp); r = r->next; } } Polylist AddPolylist()/*创建两个多项式并相加,完成后显示序列*/ {Polylist l1 = CreatePolylist(); Polylist l2 = CreatePolylist(); Polylist l3; InitPolylist(&l3); Polynode *s; Polylist r1 = l1->next, r2 = l2->next, r3 = l3; double sum; while(r1 != NULL && r2 != NULL) { s = (Polynode *)malloc(sizeof(Polynode)); if(r1->exp < r2->exp) { s->coef = r1->coef; s->exp = r1->exp; r3->next = s; r3 = s; r1 = r1->next; } else if (r1->exp == r2->exp) { sum = r1->coef + r2->coef; if(sum != 0) { s->coef = sum; s->exp = r1->exp; r3->next = s; r3 = s; r1 = r1->next; r2 = r2->next; } else { return l3; } } else { s->coef = r2->coef; s->exp = r2->exp; r3->next = s; r3 = s; r2 = r2->next; } } if(r1 != NULL){ r3->next = r1; } else { r3->next = r2; } return l3; } void ComputePolylist(Polylist L)/*计算多项式在x=%d的值*/ {Polylist r = L->next; double x = 0; double result = 0; scanf("%lf",&x); while(r != NULL) { result += r->coef * pow(x, r->exp); r = r->next; } printf("ComputePolylist in x=%.2f result is:%.2f\n",x,result); } void DestroyPolylist(Polylist L) { Polynode *p = L ,*q = L->next; while(q) { free(p); p = q; q = q->next; } free(p); printf("destroy"); } 解释一下这个代码
这段代码是一个用于处理一元多项式的链表操作程序。其中,Polylist 是一个指向 Polynode 的指针,而 Polynode 则定义了多项式中的每一个节点,包括系数和指数。程序提供了创建多项式、计算多项式值、相加多项式等功能。具体来说,CreatePolylist 函数用于输入多项式的系数和指数,用尾插法建立一元多项式的链表;AddPolylist 函数用于创建两个多项式并相加,完成后显示序列;ComputePolylist 函数用于计算多项式在给定值 x 上的值;DestroyPolylist 函数用于销毁多项式链表。该程序中的链表操作用到了常见的链表操作技巧,如链表的初始化、节点的创建、节点移动等。
相关推荐
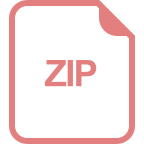
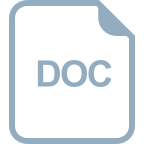












