tensorflow2 mobilenetv3
时间: 2023-05-14 21:00:22 浏览: 134
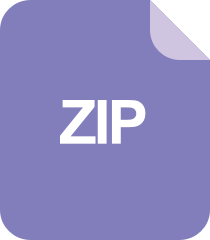
mobilenetv3_centernet:一个Tensorflow实现mobilenetv3 centernet,可以轻松地部署在android(MNN)和ios(CoreML)上
在深度学习模型中,移动设备上的模型优化是一个非常重要的话题。TensorFlow2中,MobileNetV3就是一个非常出色的解决方案。
MobileNetV3是针对移动端环境开发的,具有较轻的模型体积和快速的推理速度。与MobileNetV2相比,MobileNetV3的改进之处主要在于两个方面:1)改进了网络架构,增加了inverted residual block,使得网络更加高效;2)通过调整神经元激活函数和卷积滤波器通道数等方式,进一步优化了模型的性能。另外,MobileNetV3也支持多种损失函数和模型大小的选择,让开发者可以针对不同场景选择最适合的模型配置。
MobileNetV3最大的优势在于其快速的推理速度和较小的模型体积,这使得它非常适合在移动设备上进行物体识别和图像分类等任务,同时其精度也在不断优化。未来,MobileNetV3有望成为移动端深度学习模型优化的标准之一。
阅读全文
相关推荐
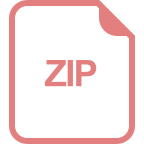
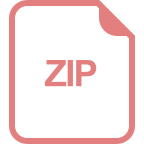















