vb.net给定一个无重复元素的数组 candidates 和一个目标数 target ,找出 candidates 中所有可以使数字和为 target 的组合。
时间: 2024-09-10 18:26:53 浏览: 45
在 VB.NET 中,你可以使用回溯算法(Backtracking)解决这个问题,这是一个典型的“零一背包”问题。以下是基本的解决方案步骤:
1. 定义一个函数 `FindCombinations`,接受两个参数:候选数组 `candidates` 和目标数 `target`。
2. 创建一个辅助函数 `IsPossible`,检查当前候选值加上已选择值是否等于目标,以及是否有剩余的候选值可以继续尝试。
3. 使用递归:开始时,设置起始点 `start = 0` 和一个空的结果列表 `result`。
4. 在主函数中调用 `FindCombinations(candidates, target, start, List(Of Integer)())`。
5. 函数内部循环遍历 `candidates`,对于每个元素,如果它与当前和相加不超过目标并且还有剩余的组合空间,就将这个元素添加到结果中,并更新当前的和和剩余的元素范围;然后递归地尝试下一个元素。
6. 返回结果列表。
```vbnet
Public Function FindCombinations(candidates As Integer(), target As Integer, start As Integer, current As List(Of Integer)) As List(Of List(Of Integer))
If start = candidates.Length Then ' 所有元素都尝试过了,如果没有达到目标,则返回空列表
Return (current.Sum() = target) And current.Count > 0 ? New List(Of List(Of Integer))(New List(Of Integer)(current)) : New List(Of List(Of Integer))()
End If
Dim result As New List(Of List(Of Integer))
For i As Integer = start To candidates.Length - 1 ' 遍历候选数组
If current.Sum() + candidates(i) <= target Then ' 如果可行,尝试加入
current.Add(candidates(i))
result.AddRange(FindCombinations(candidates, target, i + 1, current))
current.RemoveAt(current.Count - 1) ' 回溯,移除最后一个元素
End If
Next
Return result
End Function
-- 示例使用 --
Dim candidates As Integer() = {2, 7, 11, 15}
Dim target As Integer = 9
Dim combinations = FindCombinations(candidates, target, 0, New List(Of Integer)())
```
阅读全文
相关推荐
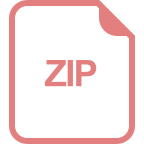
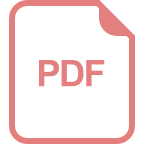
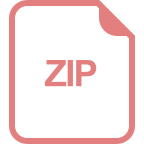
















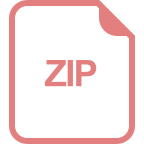