用c语言写一个,交换双向链表结点的调用函数
时间: 2024-11-09 14:30:18 浏览: 24
在C语言中,我们可以编写一个用于交换两个双向链表节点值的函数。首先,我们需要定义结构体表示链表节点,并包含前驱和后继指针。这里是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义双向链表节点结构
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
// 创建新节点的函数
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->prev = NULL;
newNode->next = NULL;
}
return newNode;
}
// 交换节点值的函数
void swapNodes(Node** head1, Node** head2) {
if (*head1 == NULL || *head2 == NULL)
return; // 检查链表是否为空
Node* temp1 = *head1;
Node* temp2 = *head2;
// 临时保存头节点的数据
int tempData1 = temp1->data;
int tempData2 = temp2->data;
// 将头节点指向对方的头节点
*head1 = (*head2)->next;
(*head1)->prev = NULL;
if (*head1 != NULL)
(*head1)->next->prev = NULL;
*head2 = temp1;
(*head2)->prev = NULL;
(*head2)->next = temp1->next;
if (*head2 != NULL)
(*head2)->next->prev = *head2;
// 交换节点数据
temp1->data = tempData2;
temp2->data = tempData1;
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* list1 = createNode(1); // 创建第一个链表
list1->next = createNode(2); // ... 等等
Node* list2 = createNode(3); // 创建第二个链表
list2->next = createNode(4);
// 假设list1的头节点是a, list2的头节点是b
Node** heads = &list1, **headsTemp = &list2;
swapNodes(heads, headsTemp); // 调用函数交换节点
// 打印交换后的链表
printf("After swapping:\n");
printList(*heads);
printList(*headsTemp);
return 0;
}
```
这个示例中,`swapNodes` 函数接受两个双向链表头节点的地址作为参数,通过一系列操作实现了节点值的交换。记得在实际应用中处理好边界条件和内存管理。
阅读全文
相关推荐
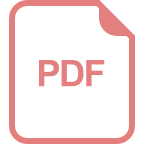
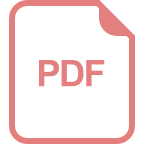
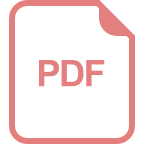



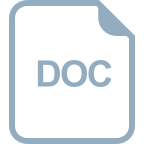












