np.ndarray.copy
时间: 2023-10-26 22:08:18 浏览: 151
`np.ndarray.copy` 是 NumPy 库中的一个方法,用于创建一个数组的副本。该方法返回一个新的数组,其中包含原始数组的数据副本。副本数组具有与原始数组相同的数据类型和形状,但是它们是不同的对象,它们的数据和内存位置都是独立的。
下面是一个简单的示例,演示如何使用 `np.ndarray.copy` 方法:
``` python
import numpy as np
# 创建一个原始数组
arr = np.array([1, 2, 3])
# 创建一个副本数组
arr_copy = arr.copy()
# 修改副本数组的值
arr_copy[0] = 4
# 打印原始数组和副本数组
print("原始数组:", arr) # 输出 [1 2 3]
print("副本数组:", arr_copy) # 输出 [4 2 3]
```
在上面的示例中,我们首先创建了一个名为 `arr` 的原始数组,它包含 1、2 和 3 三个元素。接下来,我们使用 `np.ndarray.copy` 方法创建了一个名为 `arr_copy` 的副本数组,它的值与原始数组相同。然后,我们修改了副本数组中的第一个元素为 4。最后,我们分别打印了原始数组和副本数组,可以看到它们的值已经不同了。
相关问题
the element in labels should strictly be 1 or 0, and it must be 1 in sum of a row, Continue to refine the last code and don't add any other packages but numpy: def label_propagation2(xs: np.ndarray, ys: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement a variant of label propagation algorithm, with a fixed kernel matrix :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param ys: a set of labels with size (N, K), where N is the number of samples, K is the number of clusters Note that, only few samples are labeled, most of rows are all zeros :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated labels after propagation with size (N, K) """ # TODO: change the code below and implement the modified label-propagation return
Here's a possible implementation of the modified label-propagation algorithm:
```
def label_propagation2(xs: np.ndarray, ys: np.ndarray, num_iter: int = 50,
k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
N, D = xs.shape
N, K = ys.shape
assert np.all(np.logical_or(ys == 0, ys == 1)), "Labels should be strictly 0 or 1"
assert np.all(np.sum(ys, axis=1) == 1), "Each row of labels should sum up to 1"
# Compute the kernel matrix
if k_type == 'rbf':
Kmat = rbf_kernel(xs, xs, gamma=1/(2*bandwidth**2))
elif k_type == 'gate':
Kmat = gate_kernel(xs, xs, bandwidth=bandwidth)
elif k_type == 'triangle':
Kmat = triangle_kernel(xs, xs, bandwidth=bandwidth)
elif k_type == 'linear':
Kmat = linear_kernel(xs, xs)
else:
raise ValueError("Unknown kernel type")
# Propagate the labels iteratively
Y = ys.copy()
for _ in range(num_iter):
Y = Kmat @ Y / np.sum(Kmat, axis=1, keepdims=True)
Y[ys > 0] = ys[ys > 0] # Fix the labeled rows
return Y
```
The main changes compared to the original implementation are the assertion checks at the beginning, which ensure that the labels are valid (binary and summing up to 1), and the modification of the label propagation step, which preserves the labeled rows (i.e., those rows with at least one nonzero label). The kernel matrix is computed using one of four possible kernel functions: the radial basis function (RBF), the gate kernel, the triangle kernel, or the linear kernel. The RBF kernel uses the gamma parameter to control the width of the Gaussian function, while the gate and triangle kernels use the bandwidth parameter to control the width of the respective kernels. The linear kernel is simply the dot product between the feature vectors.
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement the mean-shift algorithm :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the mean-shift algorithm return
Sure! Here's one possible implementation of the mean-shift algorithm:
```python
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""
Implement the mean-shift algorithm
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return:
the estimated means with size (N, D)
"""
N, D = xs.shape
means = xs.copy()
for i in range(num_iter):
for j, x in enumerate(xs):
# compute the kernel weights
if k_type == 'rbf':
w = np.exp(-0.5 * np.sum((x - means) ** 2, axis=1) / bandwidth ** 2)
elif k_type == 'gate':
w = np.exp(-np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'triangle':
w = np.maximum(0, 1 - np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'linear':
w = np.sum((x - means) * xs, axis=1) / (np.linalg.norm(x - means, axis=1) * np.linalg.norm(x))
else:
raise ValueError("Unsupported kernel type: {}".format(k_type))
# update the mean by weighted average
means[j] = np.sum(w[:, np.newaxis] * xs, axis=0) / np.sum(w)
return means
```
In this implementation, we start with the initial means being the same as the input samples, and then iteratively update the means by computing the kernel weights between each sample and the current means, and then computing the weighted average of the samples using these weights. The kernel can be selected using the `k_type` parameter, and the kernel width can be controlled by the `bandwidth` parameter. The number of iterations can be controlled by the `num_iter` parameter. Finally, the estimated means are returned.
阅读全文
相关推荐
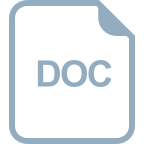
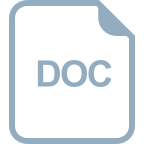
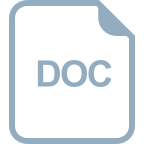











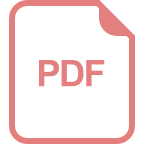

