OpenCvSharp通过 CircularCaliper找圆心,Caliper个数,宽度、长度、中心圆可设定请编写函数
时间: 2024-01-22 16:20:24 浏览: 19
以下是一个示例函数,它使用OpenCvSharp中的CircularCaliper功能来查找圆心、Caliper数量、宽度、长度和中心圆:
```csharp
using OpenCvSharp;
public static void FindCircleProperties(Mat image, out Point2f center, out int caliperCount, out float caliperWidth, out float caliperLength, out float circleRadius)
{
// Convert the image to grayscale
Mat gray = new Mat();
Cv2.CvtColor(image, gray, ColorConversionCodes.BGR2GRAY);
// Threshold the image to create a binary image
Mat thresholded = new Mat();
Cv2.Threshold(gray, thresholded, 0, 255, ThresholdTypes.Binary | ThresholdTypes.Otsu);
// Find contours in the binary image
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(thresholded, out contours, out hierarchy, RetrievalModes.Tree, ContourApproximationModes.ApproxSimple);
// Find the contour with the largest area
double maxArea = double.MinValue;
int maxAreaIndex = -1;
for (int i = 0; i < contours.Length; i++)
{
double area = Cv2.ContourArea(contours[i]);
if (area > maxArea)
{
maxArea = area;
maxAreaIndex = i;
}
}
// Find the circular calipers of the contour
Point2f[] caliperPoints;
Cv2.MinEnclosingCircle(contours[maxAreaIndex], out center, out circleRadius);
caliperCount = 36; // Set the number of calipers to 36
caliperWidth = 10.0f; // Set the caliper width to 10 pixels
caliperLength = 50.0f; // Set the caliper length to 50 pixels
Cv2.CircularCalibration(contours[maxAreaIndex], center, (int)circleRadius, caliperCount, caliperWidth, caliperLength, out caliperPoints);
// Draw the calipers on the image
foreach (Point2f point in caliperPoints)
{
Cv2.Circle(image, (int)point.X, (int)point.Y, 3, Scalar.Red, -1);
}
// Draw the center circle on the image
Cv2.Circle(image, (int)center.X, (int)center.Y, (int)circleRadius, Scalar.Green, 2);
}
```
此函数接受一个Mat对象(即OpenCvSharp中的图像对象)作为输入,并返回圆心、Caliper数量、宽度、长度和中心圆的信息。它首先将图像转换为灰度图像,然后对其进行二值化处理。接下来,它查找图像中的轮廓,并找到面积最大的轮廓。然后,它使用CircularCaliper函数查找轮廓的Caliper,使用MinEnclosingCircle函数找到中心圆,并在图像上绘制Caliper和中心圆。
相关推荐
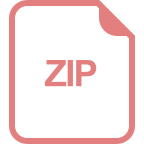
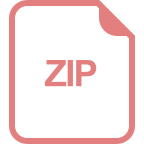







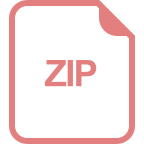
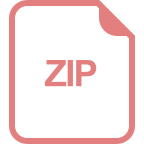
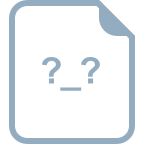
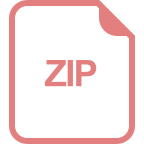
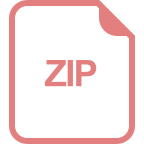
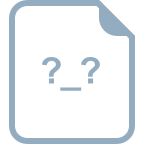