4组数据分段线性插值c语言,输出分段段曲线函数公式,输出曲线图像
时间: 2024-04-11 13:26:08 浏览: 138
要实现分段线性插值的曲线函数公式和输出曲线图像,可以使用以下的C语言代码示例:
```c
#include <stdio.h>
// 定义数据点的结构体
typedef struct {
double x;
double y;
} Point;
// 分段线性插值函数
double linearInterpolation(double x, Point* points, int numPoints) {
int i;
// 在数据点数组中找到合适的区间
for (i = 1; i < numPoints; i++) {
if (x < points[i].x) {
break;
}
}
// 计算斜率和截距
double slope = (points[i].y - points[i-1].y) / (points[i].x - points[i-1].x);
double intercept = points[i-1].y - slope * points[i-1].x;
// 计算插值结果
double interpolatedY = slope * x + intercept;
return interpolatedY;
}
int main() {
// 定义数据点数组
Point points[] = {{0.0, 0.0}, {1.0, 2.0}, {2.5, 4.5}, {4.0, 3.0}, {5.0, 5.0}};
int numPoints = sizeof(points) / sizeof(points[0]);
// 输出分段曲线函数公式
printf("分段曲线函数公式:\n");
for (int i = 1; i < numPoints; i++) {
printf("y = %.2fx + %.2f,%.2f <= x <= %.2f\n",
(points[i].y - points[i-1].y) / (points[i].x - points[i-1].x),
points[i-1].y - ((points[i].y - points[i-1].y) / (points[i].x - points[i-1].x)) * points[i-1].x,
points[i-1].x,
points[i].x);
}
// 输出曲线图像
printf("\n曲线图像:\n");
for (double x = 0.0; x <= 5.0; x += 0.2) {
double interpolatedY = linearInterpolation(x, points, numPoints);
printf("%.2f\t%.2f\n", x, interpolatedY);
}
return 0;
}
```
上述代码首先定义了一个`Point`结构体来表示数据点的坐标,然后实现了`linearInterpolation`函数来进行分段线性插值。在主函数中,我们定义了一个数据点数组`points`,然后通过调用`linearInterpolation`函数来计算给定x值的插值结果。最后,通过循环输出分段曲线函数公式和曲线图像的坐标点。
你可以根据自己的需求修改数据点数组`points`以及循环中的步长和范围,来得到你想要的分段线性插值曲线函数公式和曲线图像。
阅读全文
相关推荐


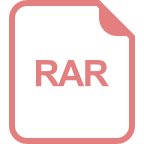
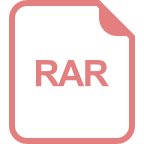
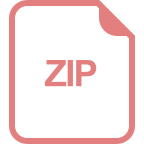
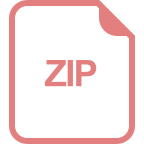
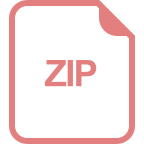
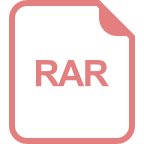
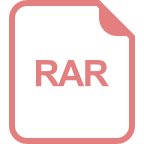
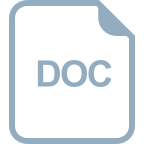
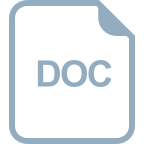
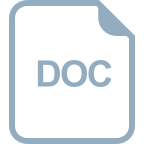
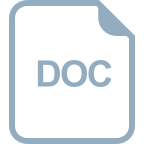
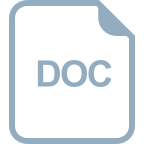

