struct b { // ... }; struct a : public b { // ... };
时间: 2024-05-10 17:14:20 浏览: 121
这段代码定义了两个结构体 `a` 和 `b` ,其中 `a` 继承自 `b`。关键字 `struct` 用于定义一个结构体。冒号 `:` 用于表示继承关系,`public` 表示继承方式为公有继承。公有继承表示 `a` 中的所有成员函数和变量都可以直接使用 `b` 中的公有成员函数和变量。例如:
```cpp
struct b {
int x;
void foo() { std::cout << "foo in b" << std::endl; }
};
struct a : public b {
int y;
void bar() { std::cout << "bar in a" << std::endl; }
};
int main() {
a obj;
obj.x = 1; // 可以直接访问 b 中的公有成员变量
obj.foo(); // 可以直接调用 b 中的公有成员函数
obj.y = 2; // a 自身的成员变量
obj.bar(); // a 自身的成员函数
return 0;
}
```
注意,结构体和类的区别是默认的成员访问权限不同。结构体默认的成员访问权限是公有的,而类默认的成员访问权限是私有的。所以上述代码中 `struct a : public b` 可以简写为 `class a : public b`。
相关问题
Traceback (most recent call last): File "/public2/tanglong/project/UNIT-master/train.py", line 39, in <module> trainer = UNIT_Trainer(config) File "/public2/tanglong/project/UNIT-master/trainer.py", line 232, in __init__ self.vgg = load_vgg16(hyperparameters['vgg_model_path'] + '/models') File "/public2/tanglong/project/UNIT-master/utils.py", line 226, in load_vgg16 vgglua = load_lua(os.path.join(model_dir, 'vgg16.t7')) File "/public/apps/anaconda3/envs/tl_unit/lib/python3.6/site-packages/torch/utils/serialization/read_lua_file.py", line 608, in load_lua return reader.read() File "/public/apps/anaconda3/envs/tl_unit/lib/python3.6/site-packages/torch/utils/serialization/read_lua_file.py", line 579, in read typeidx = self.read_int() File "/public/apps/anaconda3/envs/tl_unit/lib/python3.6/site-packages/torch/utils/serialization/read_lua_file.py", line 475, in read_int return self._read('i') File "/public/apps/anaconda3/envs/tl_unit/lib/python3.6/site-packages/torch/utils/serialization/read_lua_file.py", line 466, in _read result = struct.unpack(fmt, self.f.read(sz)) struct.error: unpack requires a buffer of 4 bytes
这个错误是由于在加载 VGG16 模型时出现了问题。根据错误信息,看起来是加载 VGG16 模型文件时发生了解析错误。这可能是由于模型文件损坏或不兼容导致的。
你可以尝试重新下载或获取一个正确的 VGG16 模型文件,然后将其替换原来的模型文件。另外,确保你的代码和模型文件版本兼容,以避免潜在的兼容性问题。
如果问题仍然存在,请检查你的文件路径是否正确,并确保你有足够的权限来读取模型文件。
希望这些信息对你有帮助!如果你还有其他问题,请随时提问。
public interface IRBNode : IComparable { IRBNode Left { get; set; } IRBNode Right { get; set; } Color Color { get; set; } IRBNode Parent { get; set; } IRBNode Grandparent(); IRBNode Sibling(); // { //#if ASSERT // Debug.Assert(Parent != null); // Root node has no sibling //#endif // if (this == Parent.Left) // return Parent.Right; // else // return Parent.Left; // } IRBNode Uncle(); // { //#if ASSERT // Debug.Assert(Parent != null); // Root node has no uncle // Debug.Assert(Parent.Parent != null); // Children of root have no uncle //#endif // return Parent.Sibling(); // } // } void AssignValueTo(IRBNode other); }转化为c
# C# 接口定义
public interface IRBNode : IComparable
{
IRBNode Left { get; set; }
IRBNode Right { get; set; }
Color Color { get; set; }
IRBNode Parent { get; set; }
IRBNode Grandparent();
IRBNode Sibling();
IRBNode Uncle();
void AssignValueTo(IRBNode other);
}
# 转化为 C 语言结构体定义
typedef struct IRBNode {
struct IRBNode* Left;
struct IRBNode* Right;
Color Color;
struct IRBNode* Parent;
struct IRBNode* (*Grandparent)();
struct IRBNode* (*Sibling)();
struct IRBNode* (*Uncle)();
void (*AssignValueTo)(struct IRBNode* other);
} IRBNode;
阅读全文
相关推荐
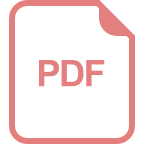
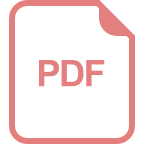













