最速下降法解方程组python
时间: 2023-06-21 17:21:48 浏览: 129
最速下降法是一种迭代法,用于解决线性方程组。下面是用Python实现最速下降法解线性方程组的示例代码:
```python
import numpy as np
# 定义线性方程组的系数矩阵A和常数向量b
A = np.array([[4, -1, 0], [-1, 4, -1], [0, -1, 4]])
b = np.array([3, 4, -5])
# 定义初始值x0和迭代次数N
x0 = np.array([0, 0, 0])
N = 50
# 定义最速下降法迭代函数
def steepestDescent(A, b, x0, N):
x = x0
for i in range(N):
r = b - np.dot(A, x) # 计算残差
alpha = np.dot(r, r) / np.dot(np.dot(A, r), r) # 计算步长
x = x + alpha * r # 更新x
return x
# 调用函数求解
x = steepestDescent(A, b, x0, N)
print(x)
```
在代码中,首先定义了线性方程组的系数矩阵A和常数向量b,然后定义了初始值x0和迭代次数N。最后定义了最速下降法迭代函数steepestDescent,其中r表示残差,alpha表示步长,x表示未知数向量。在每次迭代中,首先计算残差,然后计算步长,最后更新x。最后调用函数求解,输出结果。
相关问题
最速下降法python方程组
最速下降法是一种求解无约束优化问题的方法,可以用于求解非线性方程组。下面是使用Python实现最速下降法求解非线性方程组的示例代码:
```python
import numpy as np
def f(x):
return np.array([x[0]**2 + x[1]**2 - 1, x[0] - x[1]**3])
def df(x):
return np.array([[2*x[0], 2*x[1]], [1, -3*x[1]**2]])
def steepest_descent(x0, f, df, tol=1e-6, max_iter=100):
x = x0
for i in range(max_iter):
g = df(x).T @ f(x)
if np.linalg.norm(g) < tol:
break
d = -g
alpha = -(g.T @ d) / (d.T @ df(x) @ d)
x = x + alpha * d
return x
x0 = np.array([1, 1])
x = steepest_descent(x0, f, df)
print(x)
```
上述代码中,`f`函数表示非线性方程组,`df`函数表示`f`函数的导数,`steepest_descent`函数表示最速下降法的实现。在这个例子中,我们求解的是$x_1^2+x_2^2=1$和$x_1=x_2^3$的交点,即非线性方程组$f(x)=0$的解。
最速下降法python线性方程组
使用最速下降法解决线性方程组是一种常见的数值方法。下面是一个使用Python实现最速下降法求解线性方程组的示例代码:
```python
import numpy as np
def steepest_descent(A, b, x0, max_iter=100, tol=1e-6):
n = A.shape[0]
x = x0.copy()
r = b - np.dot(A, x)
iter_count = 0
while np.linalg.norm(r) > tol and iter_count < max_iter:
alpha = np.dot(r, r) / np.dot(np.dot(A, r), r)
x = x + alpha * r
r = b - np.dot(A, x)
iter_count += 1
return x
A = np.array([[2, 1], [1, 2]])
b = np.array([1, 2])
x0 = np.zeros_like(b)
solution = steepest_descent(A, b, x0)
print("Solution:", solution)
```
相关推荐
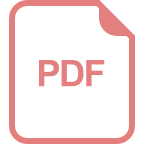
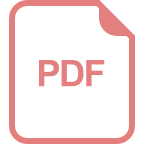
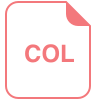
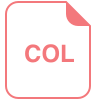
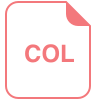
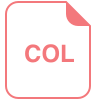
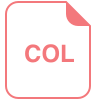







