生成用三元组实现系数矩阵的存储与运算的代码 c++
时间: 2024-02-03 08:12:51 浏览: 219
以下是用三元组实现系数矩阵的存储与运算的 C++ 代码:
```cpp
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 稀疏矩阵最大元素个数
const int ROW = 3; // 稀疏矩阵行数
const int COL = 3; // 稀疏矩阵列数
struct Matrix {
int row, col, data[MAXSIZE], pos[MAXSIZE];
} A, B, C;
void initMatrix(Matrix &matrix, int a[][COL], int row, int col) {
matrix.row = row;
matrix.col = col;
int cnt = 0;
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
if(a[i][j] != 0) {
matrix.data[cnt] = a[i][j];
matrix.pos[cnt] = i * col + j;
cnt++;
}
}
}
}
void printMatrix(Matrix matrix) {
cout << matrix.row << ' ' << matrix.col << ' ' << endl;
for(int i = 0; i < MAXSIZE; i++) {
if(matrix.data[i] != 0) {
cout << matrix.pos[i] / matrix.col << ' ' << matrix.pos[i] % matrix.col << ' ' << matrix.data[i] << endl;
}
}
}
void addMatrix(Matrix A, Matrix B, Matrix &C) {
if(A.row != B.row || A.col != B.col) {
cout << "Error: The two matrices have different dimensions." << endl;
return;
}
int cnt = 0;
for(int i = 0; i < MAXSIZE; i++) {
if(A.data[i] != 0 || B.data[i] != 0) {
C.data[cnt] = A.data[i] + B.data[i];
C.pos[cnt] = A.pos[i];
if(C.data[cnt] != 0) cnt++;
}
}
C.row = A.row;
C.col = A.col;
}
void mulMatrix(Matrix A, Matrix B, Matrix &C) {
if(A.col != B.row) {
cout << "Error: The two matrices cannot be multiplied." << endl;
return;
}
int cnt = 0;
for(int i = 0; i < A.row; i++) {
for(int j = 0; j < B.col; j++) {
int sum = 0;
for(int k = 0; k < A.col; k++) {
int a = 0, b = 0;
for(int l = 0; l < MAXSIZE; l++) {
if(A.pos[l] / A.col == i && A.pos[l] % A.col == k) {
a = A.data[l];
}
if(B.pos[l] / B.col == k && B.pos[l] % B.col == j) {
b = B.data[l];
}
}
sum += a * b;
}
if(sum != 0) {
C.data[cnt] = sum;
C.pos[cnt] = i * B.col + j;
cnt++;
}
}
}
C.row = A.row;
C.col = B.col;
}
int main() {
int a[ROW][COL] = {{1, 0, 0}, {0, 2, 0}, {0, 0, 3}};
int b[ROW][COL] = {{4, 0, 0}, {0, 5, 0}, {0, 0, 6}};
initMatrix(A, a, ROW, COL);
initMatrix(B, b, ROW, COL);
addMatrix(A, B, C);
printMatrix(C);
mulMatrix(A, B, C);
printMatrix(C);
return 0;
}
```
在这个代码中,我们定义了一个结构体 `Matrix` 来存储稀疏矩阵。其中,`row` 和 `col` 表示行数和列数,`data` 和 `pos` 分别表示矩阵元素的值和位置。在初始化矩阵时,我们遍历矩阵中的所有元素,将非零元素存储在 `data` 数组中,对应的位置存储在 `pos` 数组中。在打印矩阵时,我们只需要遍历 `data` 数组,输出非零元素的值和位置即可。
在矩阵加法和乘法运算中,我们需要遍历两个矩阵的所有元素,找到它们的对应位置,进行相应的加法或乘法运算。由于稀疏矩阵的元素大多数为零,因此在遍历时可以跳过值为零的元素。
你可以将其复制到本地编辑器中运行,以便更好地理解这个代码。
阅读全文
相关推荐


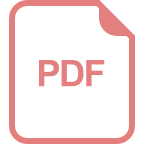
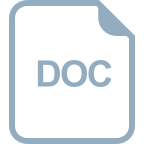
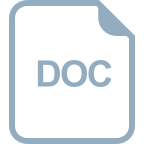
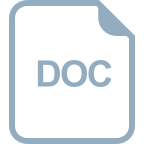
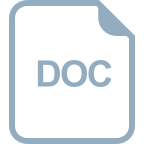
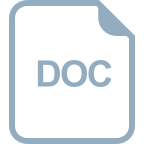
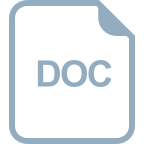
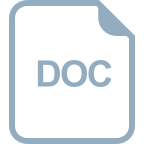
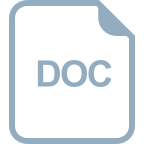
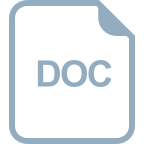
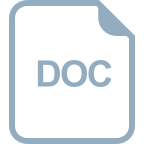

