编写程序实现折半查找算法c语言
时间: 2023-10-22 09:04:56 浏览: 49
好的,以下是实现折半查找算法的C语言代码:
```c
#include <stdio.h>
int binary_search(int arr[], int n, int target) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 11, 13};
int n = sizeof(arr) / sizeof(int);
int target = 7;
int index = binary_search(arr, n, target);
if (index != -1) {
printf("Target found at index %d\n", index);
} else {
printf("Target not found\n");
}
return 0;
}
```
在这个例子中,我们定义了一个 `binary_search` 函数来实现折半查找算法。该函数接受一个整数数组、数组长度和目标值作为参数,并返回目标值在数组中的索引。如果目标值不在数组中,则返回 -1。
在 `main` 函数中,我们定义了一个整数数组 `arr`,数组长度 `n` 和目标值 `target`。我们调用 `binary_search` 函数来查找目标值,并根据返回值输出相应的信息。在这个例子中,我们将会得到 "Target found at index 3" 的输出结果。
相关推荐
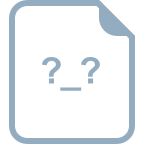














