用python 实现K-means聚类
时间: 2023-09-26 20:06:36 浏览: 194
### 回答1:
K-means 聚类是一种无监督学习算法,用于将一组数据点分成 K 个类别。下面是用 Python 实现 K-means 聚类的基本步骤:
1. 导入所需的库
```python
import numpy as np
from sklearn.cluster import KMeans
```
2. 准备数据集
```python
data = np.array([
[1, 2], [1, 4], [1, 0],
[4, 2], [4, 4], [4, 0]
])
```
3. 初始化 KMeans 对象
```python
kmeans = KMeans(n_clusters=2, random_state=0)
```
4. 训练模型
```python
kmeans.fit(data)
```
5. 获取聚类结果
```python
labels = kmeans.labels_
```
6. 获取聚类中心
```python
centroids = kmeans.cluster_centers_
```
7. 可视化聚类结果
```python
import matplotlib.pyplot as plt
plt.scatter(data[:,0], data[:,1], c=labels)
plt.scatter(centroids[:,0], centroids[:,1], marker='x', s=200, linewidths=3, color='r')
plt.show()
```
上述代码将数据集分为 2 个类别,并用红色叉号表示聚类中心。
### 回答2:
K-means聚类是一种常用的无监督学习算法,其目标是将数据集划分为K个不重叠的簇,使得簇内的数据点相似度较高,簇间的相似度较低。
首先,我们需要假设要聚类的数据点是由N个d维向量表示的,其中N表示数据点的数量,d表示每个数据点的维度。
K-means聚类的算法步骤如下:
1. 随机初始化K个簇的质心(centroids),每个簇的质心是一个d维向量。
2. 对于每个数据点,计算其与所有质心的欧氏距离,将数据点分配给距离最近的质心所对应的簇。
3. 更新每个簇的质心,即计算当前簇内所有数据点的均值,作为新的质心。
4. 重复步骤2和步骤3,直到达到停止条件(例如达到最大迭代次数,或者簇中心不再变化)。
下面是使用Python实现K-means聚类的示例代码:
```python
import numpy as np
def kmeans(X, K, max_iterations):
# 随机初始化K个簇的质心
centroids = X[np.random.choice(range(len(X)), K, replace=False)]
for _ in range(max_iterations):
# 计算每个数据点与质心的距离
distances = np.linalg.norm(X[:, np.newaxis] - centroids, axis=-1)
# 分配每个数据点到距离最近的簇
labels = np.argmin(distances, axis=1)
# 更新每个簇的质心
new_centroids = np.empty_like(centroids)
for i in range(K):
cluster_points = X[labels == i]
new_centroids[i] = np.mean(cluster_points, axis=0)
centroids = new_centroids
return labels
# 示例用法
data = np.random.rand(100, 2) # 生成100个二维随机数据点
K = 3 # 聚类的簇数
max_iterations = 100 # 最大迭代次数
labels = kmeans(data, K, max_iterations)
```
上述代码使用numpy库进行矩阵运算,首先随机初始化K个簇的质心,然后迭代计算每个数据点与质心的距离,分配数据点到距离最近的簇,并更新每个簇的质心,直到迭代结束。最后返回聚类结果labels,即每个数据点所属的簇的标签。
注意,K-means聚类算法的实现还有一些改进和优化的方法(如K-means++初始化、二分K-means等),上述代码仅提供了基本的实现思路。
### 回答3:
K-means聚类是一种常用的无监督学习算法,用于将数据集划分为K个不同的簇。下面是使用Python实现K-means聚类的具体过程:
1. 导入必要的库:
```python
import numpy as np
import matplotlib.pyplot as plt
```
2. 定义K-means类:
```python
class KMeans:
def __init__(self, k):
self.k = k
def fit(self, X):
# 初始化聚类中心
centroid_indices = np.random.choice(range(X.shape[0]), size=self.k, replace=False)
centroids = X[centroid_indices]
while True:
# 计算每个样本与聚类中心的距离
distances = np.linalg.norm(X[:, np.newaxis, :] - centroids, axis=-1)
# 分配数据点到最近的聚类中心
labels = np.argmin(distances, axis=-1)
# 更新聚类中心
new_centroids = np.array([X[labels == i].mean(axis=0) for i in range(self.k)])
# 判断是否达到收敛
if np.all(centroids == new_centroids):
break
centroids = new_centroids
# 返回聚类结果
return labels
```
3. 导入数据集:
```python
X = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 4], [4, 0]])
```
4. 创建K-means对象并进行聚类:
```python
kmeans = KMeans(k=2)
labels = kmeans.fit(X)
```
5. 可视化聚类结果:
```python
plt.scatter(X[:, 0], X[:, 1], c=labels)
plt.show()
```
这样就完成了使用Python实现K-means聚类的过程。通过计算样本与聚类中心的距离并不断更新聚类中心,最终实现了数据集的聚类。
阅读全文
相关推荐
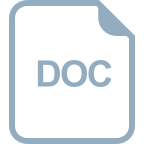
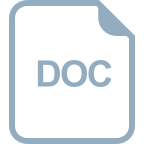
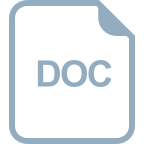
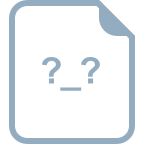
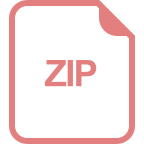
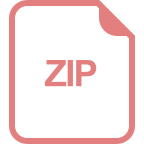
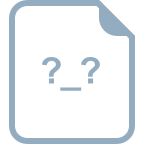
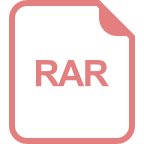
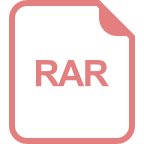
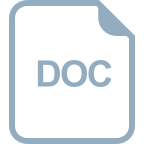
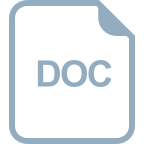
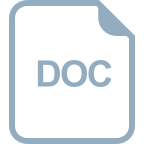





