建立二叉树时,若子树不为空,则输入的数据是整型数据,若子树为空,输入为null,该怎么写程序建立二叉树,用户输入的“null”怎么处理
时间: 2024-11-04 08:10:36 浏览: 15
在建立二叉树的递归算法中,你可以采用这样的思路:
```python
class Node:
def __init__(self, data):
self.left = None
self.right = None
self.data = data
def build_binary_tree():
# 获取用户输入
user_input = input("请输入节点值(非空输入整数,输入 'null' 结束构建):")
# 将输入转化为Node对象或None处理
if user_input == 'null':
return None
else:
data = int(user_input)
new_node = Node(data)
# 如果有左孩子,继续递归构建左子树
left_child = build_binary_tree()
new_node.left = left_child
# 如果有右孩子,继续递归构建右子树
right_child = build_binary_tree()
new_node.right = right_child
return new_node
# 主函数
root = build_binary_tree()
if root is not None:
print("二叉树构建完成,根节点为:", root.data)
else:
print("构建结束,树为空")
```
在这个程序中,当用户输入`'null'`时,我们返回`None`,表示当前没有子节点。然后我们会继续询问下一次的输入,直到用户输入结束。如果树非空,我们将创建一个`Node`实例并继续递归地构建左右子树。
阅读全文
相关推荐
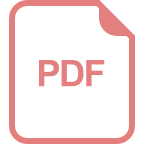
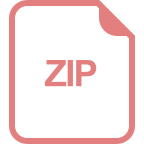
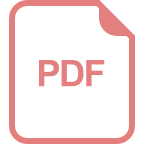



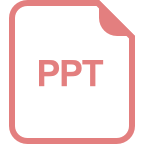
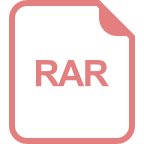
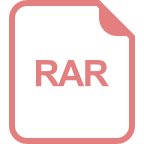
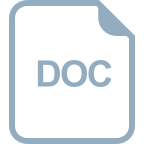
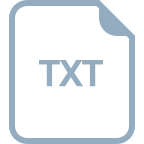







