设计程序,按先序创建二叉树的二叉链表;然后先序、中序、后序遍历二叉树。 输入格式: 按先序输入一棵二叉树。二叉树中每个结点的键值用字符表示,字符之间不含空格。注意空树信息也要提供,以#字符表示空树。 输出格式: 输出3行。第一行是先序遍历二叉树的序列,第二行是中序遍历二叉树的序列,第三行是后序遍历二叉树的序列。每行首尾不得有多余空格。序列中不含#。用C语言
时间: 2024-03-15 10:46:29 浏览: 116
以下是 C 代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct TreeNode {
char val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
TreeNode *build_tree(char *preorder, int *index) {
char val = preorder[(*index)++];
if (val == '#') {
return NULL;
}
TreeNode *root = (TreeNode *) malloc(sizeof(TreeNode));
root->val = val;
root->left = build_tree(preorder, index);
root->right = build_tree(preorder, index);
return root;
}
void preorder_traversal(TreeNode *root, char *res, int *index) {
if (root == NULL) {
return;
}
res[(*index)++] = root->val;
preorder_traversal(root->left, res, index);
preorder_traversal(root->right, res, index);
}
void inorder_traversal(TreeNode *root, char *res, int *index) {
if (root == NULL) {
return;
}
inorder_traversal(root->left, res, index);
res[(*index)++] = root->val;
inorder_traversal(root->right, res, index);
}
void postorder_traversal(TreeNode *root, char *res, int *index) {
if (root == NULL) {
return;
}
postorder_traversal(root->left, res, index);
postorder_traversal(root->right, res, index);
res[(*index)++] = root->val;
}
int main() {
char preorder[100];
scanf("%s", preorder);
int index = 0;
TreeNode *root = build_tree(preorder, &index);
char res[100];
int res_index = 0;
preorder_traversal(root, res, &res_index);
res[res_index] = '\0';
printf("%s\n", res);
res_index = 0;
inorder_traversal(root, res, &res_index);
res[res_index] = '\0';
printf("%s\n", res);
res_index = 0;
postorder_traversal(root, res, &res_index);
res[res_index] = '\0';
printf("%s\n", res);
return 0;
}
```
首先定义了一个 `TreeNode` 结构体表示二叉树节点,包含节点值和左右子节点。然后实现了 `build_tree` 函数,输入先序遍历序列和一个整型指针,递归构建二叉树。在递归过程中,每次取出先序遍历序列的一个值,如果是 `#` 表示空节点,返回 `NULL`。否则,创建一个新节点,值为当前值,然后递归构建左右子树。最后返回根节点。
接下来是三个遍历函数。先序遍历函数 `preorder_traversal` 先访问根节点,然后递归遍历左子树和右子树。中序遍历函数 `inorder_traversal` 先递归遍历左子树,然后访问根节点,最后递归遍历右子树。后序遍历函数 `postorder_traversal` 先递归遍历左子树和右子树,最后访问根节点。这三个函数都接受一个指向结果字符串和一个整型指针,用于保存遍历结果和当前结果字符串的索引。
最后读入先序遍历序列,构建二叉树,分别输出三种遍历序列即可。
阅读全文
相关推荐

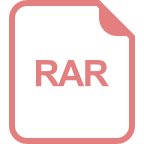













