initialization of int(*)[(sizetype)
时间: 2024-11-13 11:23:48 浏览: 8
初始化像 `int(*)[(size_t)]` 这样的表达式通常涉及到指针数组的动态内存分配。在这里,`int(*)` 表示指向整数的指针,而 `[(size_t)]` 则表示该指针可以存储任意大小的整数,由 `size_t` 类型的值决定其长度。
这种形式的初始化意味着你在创建一个动态数组,其中元素的大小是根据运行时指定的 `size_t` 值来确定的。例如:
```c++
size_t arraySize = sizeof(int); // 或者其他动态计算的大小
int (*dynamicArray)[arraySize] = malloc(arraySize * sizeof(*dynamicArray));
```
这里的 `malloc()` 函数会返回一个指针,它指向一个能容纳 `arraySize` 个 `int` 类型元素的数组。然后你可以通过下标操作符 `[]` 来访问和修改这个动态数组的元素。
然而,需要注意的是,这样的用法可能会导致内存溢出的风险,因为如果 `size_t` 的值过大,超出实际可用内存范围,就会出现问题。同时,忘记释放动态内存可能导致内存泄漏。
相关问题
initialization of 'int (*)[(sizetype)(x_len)]' from incompatible pointer type 'int * ( Wincompatible-pointer-types]
初始化 `int (*)[(size_t)(x_len)]` 从不兼容的指针类型 `int *`,实际上是指尝试将一个指向单个 `int` 变量的指针转换成一个可以存储动态数组的指针,其中数组长度取决于变量 `x_len` 的大小。但在 C++ 中,这种转换通常是不允许的,因为它们代表了不同级别的内存管理和操作。
`int *` 指向的是单个 `int` 类型的元素,而 `int (*)[(size_t)(x_len)]` 实际上是在描述一个动态数组,它可以包含任意数量的 `int` 元素,直到指定的 `x_len` 为止。所以,如果你试图这样做,编译器会报错,指出类型不匹配,因为不能直接把一个指向单一元素的指针赋给一个可以存储多元素的指针类型。
如果你需要在 C++ 中创建一个动态数组,应该明确地声明并初始化,如使用 `new []` 关键字来分配内存:
```cpp
size_t x_len;
// ... 初始化 x_len ...
int* dynamicArray = new int[x_len];
```
在这种情况下,`new int[x_len]` 会返回一个指向 `int` 类型数组的新指针,可以直接用来存储 `x_len` 个 `int` 元素。
#include "redismanager.h" #include <QDebug> RedisManager::RedisManager(QObject* parent) : QObject(parent) { context = redisConnect("127.0.0.1", 6379); if (context == NULL || context->err) { if (context) { qDebug() << "Error: " << context->errstr; redisFree(context); } else { qDebug() << "Can't allocate redis context"; } } } void RedisManager::getFirstLayerKeys() { QSet<QString> firstLayer; QJsonObject keyInfos; QString cursor = "0"; do { redisReply* reply = (redisReply*)redisCommand(context,"SCAN %s", cursor.toStdString().c_str()); if( reply == NULL ) { qDebug() << "Failed to execute command."; redisFree(context); return; } if( reply->type == REDIS_REPLY_ARRAY ) { cursor = QString(reply->element[0]->str); redisReply* keysReply = reply->element[1]; for(size_t i = 0; i < keysReply->elements; i++) { QString key = QString(keysReply->element[i]->str); int pos = key.indexOf(':'); if (pos != -1) { QString firstLayerKey = key.left(pos); if (!firstLayer.contains(firstLayerKey)) { firstLayer.insert(firstLayerKey); redisReply* countReply = (redisReply*)redisCommand(context,"KEYS %s:*", firstLayerKey.toStdString().c_str()); if (countReply->type == REDIS_REPLY_ARRAY) { keyInfos.insert(firstLayerKey, QJsonValue((int)countReply->elements)); } freeReplyObject(countReply); } } } } freeReplyObject(reply); } while (cursor != "0"); emit firstLayerKeysReady(keyInfos); }
以下是一些可能的代码优化建议:
1. 建议使用 RAII(Resource Acquisition Is Initialization)技术来管理 Redis 连接,以确保在函数退出时正确释放连接。可以使用 C++11 中的 std::unique_ptr 来管理 redisContext 对象。
2. 建议使用 Redis 的 SCAN 命令来遍历所有的 key,而不是使用 KEYS 命令。因为 KEYS 命令在 Redis 中是一个非常耗费资源的命令,而 SCAN 命令则是一种更加高效的方式来遍历所有的 key。
3. 建议在函数开始时使用 redisAppendCommand 函数来批量执行 Redis 命令,而不是在循环中使用 redisCommand 函数执行单个命令。这样可以减少网络往返次数,提高程序的性能。
4. 建议将 QSet<QString> firstLayer 和 QJsonObject keyInfos 改为成员变量,以避免在每次函数调用时重新创建和初始化。
5. 建议使用 C++11 中的 auto 关键字来自动推断变量类型,以简化代码。
下面是应用上述优化后的代码示例:
```
#include "redismanager.h"
#include <QDebug>
#include <memory>
RedisManager::RedisManager(QObject* parent) : QObject(parent) {
context = std::unique_ptr<redisContext, decltype(&redisFree)>(redisConnect("127.0.0.1", 6379), redisFree);
if (context == NULL || context->err) {
if (context) {
qDebug() << "Error: " << context->errstr;
} else {
qDebug() << "Can't allocate redis context";
}
}
}
void RedisManager::getFirstLayerKeys() {
auto firstLayer = QSet<QString>();
auto keyInfos = QJsonObject();
auto cursor = "0";
auto cmds = QStringList();
do {
cmds << QString("SCAN %1").arg(cursor);
auto reply = std::unique_ptr<redisReply, decltype(&freeReplyObject)>(nullptr, freeReplyObject);
if (redisAppendCommand(context.get(), cmds.last().toStdString().c_str()) != REDIS_OK ||
redisGetReply(context.get(), (void**)&reply) != REDIS_OK ||
reply == nullptr || reply->type != REDIS_REPLY_ARRAY || reply->elements != 2) {
qDebug() << "Failed to execute command.";
return;
}
cursor = QString(reply->element[0]->str);
auto keysReply = reply->element[1];
for (size_t i = 0; i < keysReply->elements; i++) {
auto key = QString(keysReply->element[i]->str);
auto pos = key.indexOf(':');
if (pos != -1) {
auto firstLayerKey = key.left(pos);
if (!firstLayer.contains(firstLayerKey)) {
firstLayer.insert(firstLayerKey);
cmds << QString("KEYS %1:*").arg(firstLayerKey);
}
}
}
} while (cursor != "0");
auto reply = std::unique_ptr<redisReply, decltype(&freeReplyObject)>(nullptr, freeReplyObject);
for (auto cmd : cmds) {
if (redisAppendCommand(context.get(), cmd.toStdString().c_str()) != REDIS_OK) {
qDebug() << "Failed to execute command.";
return;
}
}
for (size_t i = 0; i < cmds.size(); i++) {
if (redisGetReply(context.get(), (void**)&reply) != REDIS_OK || reply == nullptr) {
qDebug() << "Failed to execute command.";
return;
}
auto firstLayerKey = cmds[i].mid(4, cmds[i].indexOf(':') - 4);
if (reply->type == REDIS_REPLY_ARRAY) {
keyInfos.insert(firstLayerKey, QJsonValue((int)reply->elements));
}
freeReplyObject(reply);
}
emit firstLayerKeysReady(keyInfos);
}
```
阅读全文
相关推荐
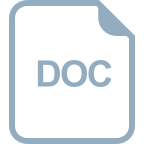
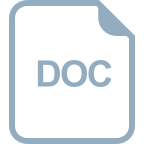
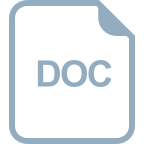
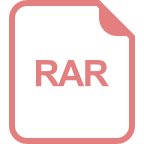
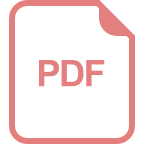
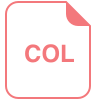
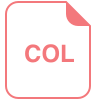
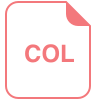
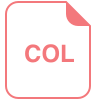
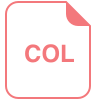
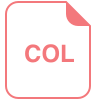
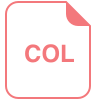
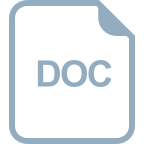
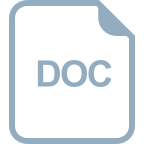