定义一个复数类,并为其重载>>和<<运算符,使得复数对象可以整体输入输出。
时间: 2024-12-24 10:19:11 浏览: 0
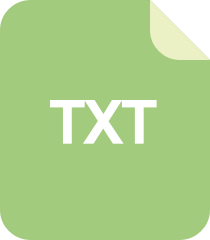
C++写的复数类设计计算
在许多编程语言中,包括C++和Python,你可以定义一个复数类来表示复数并重载`+`, `-`, `*`, `/`等基本算术运算符。对于输入输出,虽然不能直接重载`>>`和`<<`操作符(因为它们通常用于文件I/O),但我们可以通过覆盖`toString()`和`fromString()`方法来模拟这种功能。
首先,我们假设你在C++中创建一个复数类`Complex`:
```cpp
#include <iostream>
#include <string>
class Complex {
private:
double real;
double imag;
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 重载操作符实现加法、减法、乘法和除法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
// 重载to_string()和from_string()方法
std::string toString() const {
return "(" + std::to_string(real) + " + " + std::to_string(imag) + "i)";
}
static Complex fromString(const std::string& str) {
if (str.find('(') != 0 || str.rfind('i') == str.length()) {
throw std::invalid_argument("Invalid complex number format");
}
std::istringstream iss(str.substr(1, str.length() - 2));
double real, imag;
iss >> real >> imag;
return Complex(real, imag);
}
friend std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.toString();
return os;
}
};
```
在这个例子中,`operator<<`允许我们将复数对象输出到标准流,而`fromString`方法则可以解析形如`(a + bi)`的字符串。注意,真正的`>>`操作符在这里没有被重载,因为它主要用于控制台输入,如果你需要支持从文件中读取复数,你需要另外处理。
阅读全文
相关推荐
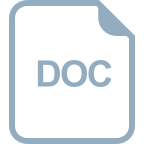
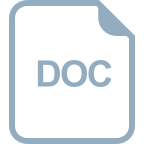















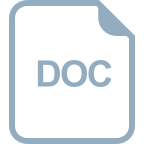