python四元数tfs
时间: 2023-10-17 16:27:37 浏览: 71
Python中处理四元数的库是quaternion。可以使用该库进行四元数的创建、旋转、插值等操作。下面是一个使用quaternion库进行四元数变换的示例代码:
```python
import numpy as np
import quaternion
# 创建四元数
q1 = np.quaternion(1, 2, 3, 4)
q2 = np.quaternion(5, 6, 7, 8)
# 四元数乘法
q_mul = q1 * q2
# 四元数共轭
q_conj = np.conjugate(q1)
# 四元数旋转
v = np.quaternion(0, 1, 0, 0) # 要旋转的向量
rotated_v = q1 * v * q_conj
print("q_mul:", q_mul)
print("q_conj:", q_conj)
print("rotated_v:", rotated_v)
```
需要注意的是,quaternion库中的四元数遵循以下规则:q = w + xi + yj + zk,其中w、x、y、z都是实数。在该库中,四元数可以直接进行乘法和共轭操作,并且可以与向量进行旋转运算。
相关问题
python四元数解算姿态角
四元数是一种用于表示旋转姿态的数学工具,它可以用来描述物体在三维空间中的旋转。在Python中,可以使用`quaternion`库来进行四元数的解算和姿态角的计算。
首先,你需要安装`quaternion`库。可以使用以下命令来安装:
```
pip install numpy-quaternion
```
安装完成后,你可以使用以下代码来进行四元数的解算和姿态角的计算:
```python
import numpy as np
from numpy import quaternion
# 创建一个四元数对象
q = quaternion.from_rotation_vector([0.1, 0.2, 0.3])
# 将四元数转换为旋转矩阵
rotation_matrix = quaternion.as_rotation_matrix(q)
# 将旋转矩阵转换为欧拉角(姿态角)
euler_angles = np.degrees(quaternion.as_euler_angles(q))
print("四元数:", q)
print("旋转矩阵:", rotation_matrix)
print("欧拉角(姿态角):", euler_angles)
```
在上面的代码中,我们首先创建了一个四元数对象`q`,然后使用`quaternion.as_rotation_matrix()`函数将其转换为旋转矩阵,最后使用`quaternion.as_euler_angles()`函数将旋转矩阵转换为欧拉角(姿态角)。输出结果分别为四元数、旋转矩阵和欧拉角。
希望以上信息对你有帮助!如果你有任何其他问题,请随时提问。
python四元数转欧拉角的代码
### 回答1:
答:可以使用以下代码实现python四元数转欧拉角:from math import atan2,asin,pidef quaternion_to_euler(w, x, y, z):
ysqr = y * y
t0 = +2.0 * (w * x + y * z)
t1 = +1.0 - 2.0 * (x * x + ysqr)
X = atan2(t0, t1)
t2 = +2.0 * (w * y - z * x)
t2 = +1.0 if t2 > +1.0 else t2
t2 = -1.0 if t2 < -1.0 else t2
Y = asin(t2)
t3 = +2.0 * (w * z + x * y)
t4 = +1.0 - 2.0 * (ysqr + z * z)
Z = atan2(t3, t4)
return X, Y, Z
### 回答2:
要将四元数转换为欧拉角,可以使用Python中的math库中的函数进行计算。下面是一个实现四元数转为欧拉角的简单代码示例:
```python
import math
def quaternion_to_euler(q):
# 计算四元数的各个分量
w, x, y, z = q
# 计算欧拉角的各个分量
roll = math.atan2(2*(w*x + y*z), 1 - 2*(x*x + y*y))
pitch = math.asin(2*(w*y - z*x))
yaw = math.atan2(2*(w*z + x*y), 1 - 2*(y*y + z*z))
# 将弧度转换为角度
roll = math.degrees(roll)
pitch = math.degrees(pitch)
yaw = math.degrees(yaw)
# 返回欧拉角
return roll, pitch, yaw
# 示例四元数
quaternion = [0.5, 0.5, 0.5, 0.5]
# 调用函数将四元数转为欧拉角
roll, pitch, yaw = quaternion_to_euler(quaternion)
# 输出结果
print("Roll: ", roll)
print("Pitch: ", pitch)
print("Yaw: ", yaw)
```
以上代码实现了将四元数转换为欧拉角的功能。其中,四元数的分量w、x、y、z通过参数q传入函数quaternion_to_euler,并在函数内部进行计算。最后,将计算得到的弧度转换为角度,并将欧拉角roll、pitch、yaw返回。在示例中使用了四元数[0.5, 0.5, 0.5, 0.5]进行转换,并打印输出结果。
### 回答3:
要将Python中的四元数转换为欧拉角,您可以使用quaternion模块中的函数。以下是一个示例代码:
```python
import math
import numpy as np
import quaternion
def quaternion_to_euler_angle(q):
# 将四元数转换为旋转矩阵
rotation_matrix = quaternion.as_rotation_matrix(q)
# 从旋转矩阵中提取欧拉角
# 使用numpy的arctan2函数计算yaw角
yaw = math.atan2(rotation_matrix[1, 0], rotation_matrix[0, 0])
# 使用numpy的arcsin函数计算pitch角
sin_pitch = -rotation_matrix[2, 0]
cos_pitch = np.sqrt(rotation_matrix[2, 1]**2 + rotation_matrix[2, 2]**2)
pitch = math.atan2(sin_pitch, cos_pitch)
# 使用numpy的arctan2函数计算roll角
roll = math.atan2(rotation_matrix[2, 1], rotation_matrix[2, 2])
# 将弧度转换为角度
yaw_deg = math.degrees(yaw)
pitch_deg = math.degrees(pitch)
roll_deg = math.degrees(roll)
return [yaw_deg, pitch_deg, roll_deg]
# 示例使用
q = quaternion.from_rotation_vector([0.1, 0.2, -0.3])
euler_angles = quaternion_to_euler_angle(q)
print("欧拉角:", euler_angles)
```
在示例中,我们首先导入必要的模块,然后定义了一个`quaternion_to_euler_angle`函数,该函数接受一个四元数作为输入并返回对应的欧拉角。函数内部使用`quaternion.as_rotation_matrix`函数将四元数转换为旋转矩阵,然后从旋转矩阵中提取欧拉角。最后,我们通过调用示例来演示函数的使用,并打印出转换后的欧拉角。
相关推荐
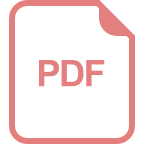
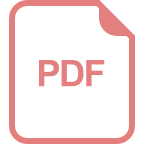












