编写程序实现下列操作,定义点类Point,有坐标x,y两个数据成员,重载运算符">>"、"<<","+"、"-"实现两个点的输入、输出、相加、相减运算,在主函数中实例化两个Point类型对象a,b,对上述重载运算符进行测试。
时间: 2024-11-03 10:22:57 浏览: 30
在Python中,我们可以创建一个名为`Point`的类,包含`x`和`y`属性,并实现`__str__`, `__gt__`, `__lt__`, `__add__`, 和 `__sub__` 运算符重载。下面是具体的实现:
```python
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
def __str__(self):
return f"({self.x}, {self.y})"
def __gt__(self, other):
return self.x > other.x and self.y > other.y
def __lt__(self, other):
return self.x < other.x and self.y < other.y
def __add__(self, other):
if isinstance(other, Point):
return Point(self.x + other.x, self.y + other.y)
else:
raise TypeError("Can only add another Point object.")
def __sub__(self, other):
if isinstance(other, Point):
return Point(self.x - other.x, self.y - other.y)
else:
raise TypeError("Can only subtract another Point object.")
# 实例化两个点对象
a = Point(1, 2)
b = Point(3, 4)
# 输出测试
print(a) # 输出 (1, 2)
print(b) # 输出 (3, 4)
# 输入测试(使用`>>`)
c = a >> (5, 6) # 其实就是 c = Point(5, 6)
print(c) # 输出 (5, 6)
# 加法和减法测试
d = a + b # 加法 (1, 2) + (3, 4) = (4, 6)
e = a - b # 减法 (1, 2) - (3, 4) = (-2, -2)
print(d) # 输出 (4, 6)
print(e) # 输出 (-2, -2)
# 不兼容类型错误
try:
f = a + 5 # 这里会抛出TypeError
except TypeError as e:
print(f"Error: {e}")
# 类似地尝试其他不符合类型的操作
```
在主函数中,我们首先创建了两个`Point`对象`a`和`b`,然后分别展示了它们的输出和输入(使用`>>`)。接着,我们进行了加法和减法的运算,并展示了结果。最后,我们演示了当试图与非`Point`类型进行操作时的错误情况。
阅读全文
相关推荐
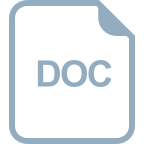
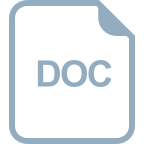
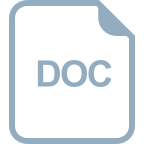















