二叉链表求二叉树中的结点数量
时间: 2024-03-01 13:46:35 浏览: 83
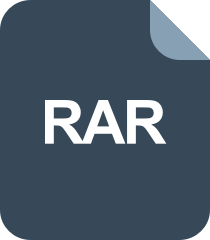
计算二叉树的结点个数

二叉链表是一种用链表表示二叉树的方法,每个结点包含一个数据元素和两个指针,分别指向左子树和右子树。要求二叉链表中的结点数量,可以通过遍历二叉链表并计数的方式来实现。
以下是一个示例代码,用于求解二叉链表中的结点数量:
```python
class Node:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
def count_nodes(root):
if root is None:
return 0
else:
return 1 + count_nodes(root.left) + count_nodes(root.right)
# 构建二叉链表
# 这里以先序序列为例,构建一个二叉链表
def build_binary_tree(preorder):
if len(preorder) == 0:
return None
else:
data = preorder.pop(0)
if data == '0':
return None
else:
node = Node(data)
node.left = build_binary_tree(preorder)
node.right = build_binary_tree(preorder)
return node
# 测试代码
preorder = ['A', 'B', 'D', '0', '0', 'E', '0', '0', 'C', 'F', '0', '0', 'G', '0', '0']
root = build_binary_tree(preorder)
node_count = count_nodes(root)
print("The number of nodes in the binary tree is:", node_count)
```
运行以上代码,可以得到二叉链表中的结点数量。在这个例子中,二叉链表中的结点数量为7。
阅读全文
相关推荐















