使用Java语言写出工厂模式的代码。(注:工厂模式分为:简单工厂模式、工厂方法模式和抽象工厂模式。这3种工厂模式都要写出代码!)
时间: 2024-11-17 19:12:55 浏览: 2
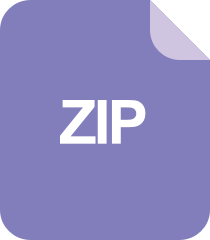
设计模式之工厂模式详细
当然可以。以下是三种常见的工厂模式的Java代码示例:
1. **简单工厂模式** (也称为裸工厂模式):
```java
// 抽象产品接口
public interface Product {
void doSomething();
}
// 具体产品A
public class ConcreteProductA implements Product {
@Override
public void doSomething() {
System.out.println("Doing something with ConcreteProductA");
}
}
// 简单工厂类
public class SimpleFactory {
public static Product createProduct(String type) {
if ("A".equals(type)) {
return new ConcreteProductA();
} else {
throw new IllegalArgumentException("Unsupported product type: " + type);
}
}
}
```
使用工厂创建产品:
```java
public class Client {
public static void main(String[] args) {
Product product = SimpleFactory.createProduct("A"); // 创建产品A
product.doSomething(); // 调用doSomething方法
}
}
```
2. **工厂方法模式**:
```java
// 抽象产品接口
public interface Product {
void doSomething();
}
// 具体产品接口
public abstract class AbstractProduct {
protected String name;
// 构造函数接收产品名称
public AbstractProduct(String name) {
this.name = name;
}
// 抽象工厂方法
public abstract void doSomething();
}
// 具体产品A实现
public class ConcreteProductA extends AbstractProduct {
public ConcreteProductA(String name) {
super(name);
}
@Override
public void doSomething() {
System.out.println("Doing something with ConcreteProductA: " + name);
}
}
// 工厂类
public class FactoryMethodFactory {
public Product createProduct(String type) {
if ("A".equals(type)) {
return new ConcreteProductA("Product A");
} else {
throw new IllegalArgumentException("Unsupported product type: " + type);
}
}
}
```
3. **抽象工厂模式**:
```java
// 抽象产品接口
public interface ProductInterface {
void doSomething();
}
// 具体产品A
public class ConcreteProductA implements ProductInterface {
@Override
public void doSomething() {
System.out.println("Doing something with ConcreteProductA");
}
}
// 抽象工厂接口
public interface FactoryInterface {
ProductInterface getProduct(String type);
}
// 具体工厂A
public class ConcreteFactoryA implements FactoryInterface {
@Override
public ProductInterface getProduct(String type) {
if ("A".equals(type)) {
return new ConcreteProductA();
} else {
throw new IllegalArgumentException("Unsupported product type: " + type);
}
}
}
// 客户端代码
public class AbstractClient {
private FactoryInterface factory;
public AbstractClient(FactoryInterface factory) {
this.factory = factory;
}
public void run() {
ProductInterface product = factory.getProduct("A");
product.doSomething();
}
}
// 主程序
public class Main {
public static void main(String[] args) {
AbstractClient client = new AbstractClient(new ConcreteFactoryA());
client.run();
}
}
```
阅读全文
相关推荐
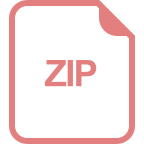
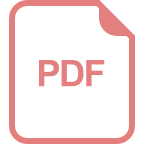















