class srmConv2d(nn.Conv2d): def __init__( self, in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, v_th=1.0, taum=5., taus=3., taug=2.5 ) -> None: super().__init__(in_channels, out_channels, kernel_size, stride, padding, dilation, groups, bias=False) nn.init.orthogonal_(self.weight) self.taum = taum self.taus = taus self.taug = taug self.v_th = v_th self.epsw = None self.epst = None self.e_taum = 1. - 1. / taum self.e_taus = 1. - 1. / taus self.e_taug = 1. - 1. / taug self.conv_func = srmConvFunc.apply def batch_reset(self, inputs: Tensor) -> None: if self.epsw is None or self.epsw.shape[0] != inputs.shape[1]: coefficient = self.taum / (self.taum - self.taus) # for i in range(inputs.shape[1]): self.epst = torch.FloatTensor([-self.e_taug ** (1 + i) for i in range(inputs.shape[1])]).to(inputs) self.epsw = torch.FloatTensor( [coefficient * (self.e_taum ** (1 + i) - self.e_taus ** (1 + i)) for i in range(inputs.shape[1])] ).to(inputs) def forward(self, inputs): self.batch_reset(inputs) return self.conv_func( inputs, self.weight, self.taum, self.taus, self.e_taug, self.v_th, self.epsw, self.epst, self.stride, self.padding, self.dilation, self.groups )
时间: 2023-12-09 20:03:28 浏览: 182
这是一个自定义的卷积层类 srmConv2d,继承自 PyTorch 自带的 nn.Conv2d。它的初始化函数中有一些额外的参数,包括 v_th、taum、taus、taug,分别表示神经元的阈值、膜电位时间常数、突触前电位时间常数和突触后电位时间常数。该类还定义了一个 batch_reset 方法,用于根据输入数据重新计算权重的一些参数,如 epst 和 epsw。最后,类中的 forward 方法调用了自定义的卷积函数 srmConvFunc.apply,该函数实现了 SRM 卷积的计算过程。
相关问题
class GhostModule(nn.Module): def __init__(self, input_channels, output_channels, kernel_size=1, ratio=2): super(GhostModule, self).__init__() self.output_channels = output_channels self.hidden_channels = output_channels // ratio self.primary_conv = nn.Sequential( nn.Conv2d(input_channels, self.hidden_channels, kernel_size, bias=False), nn.BatchNorm2d(self.hidden_channels), nn.ReLU(inplace=True) ) self.cheap_operation = nn.Sequential( nn.Conv2d(self.hidden_channels, self.hidden_channels, kernel_size, groups=self.hidden_channels, bias=False), nn.BatchNorm2d(self.hidden_channels), nn.ReLU(inplace=True) ) self.secondary_conv = nn.Sequential( nn.Conv2d(self.hidden_channels, self.output_channels - self.hidden_channels, kernel_size, bias=False), nn.BatchNorm2d(self.output_channels - self.hidden_channels) ) def forward(self, x): x1 = self.primary_conv(x) x2 = self.cheap_operation(x1) x3 = self.secondary_conv(torch.cat([x1, x2], dim=1)) return torch.cat([x1, x3], dim=1)
这是一个 PyTorch 的神经网络模块,该模块名为 GhostModule。该模块包含三个卷积层:主要卷积层、廉价卷积操作和次要卷积层。其中主要卷积层是一个标准的卷积操作,廉价卷积操作是一种深度可分离卷积,次要卷积层是一个标准的卷积操作。这个模块的目的是在减少计算成本的同时保持模型的准确性。你有没有其他问题呢?
class SelfAttention(nn.Module): def __init__(self,in_c,out_c,fm_sz,pos_bias = False): super(SelfAttention,self).__init__() self.w_q = nn.Conv2d(in_channels = in_c,out_channels = out_c,kernel_size = 1) self.w_k = nn.Conv2d(in_channels = in_c,out_channels = out_c,kernel_size = 1) self.w_v = nn.Conv2d(in_channels = in_c,out_channels = out_c,kernel_size = 1) self.pos_code = self.__getPosCode(fm_sz,out_c) self.softmax = nn.Softmax(dim = 2) self.pos_bias = pos_bias 改写为twensorflow形式
可以将这段PyTorch代码改写为如下的TensorFlow代码:
```python
import tensorflow as tf
from tensorflow import keras
class SelfAttention(keras.layers.Layer):
def __init__(self, in_c, out_c, fm_sz, pos_bias=False):
super(SelfAttention, self).__init__()
self.w_q = keras.layers.Conv2D(filters=out_c, kernel_size=1, input_shape=(None, None, in_c))
self.w_k = keras.layers.Conv2D(filters=out_c, kernel_size=1, input_shape=(None, None, in_c))
self.w_v = keras.layers.Conv2D(filters=out_c, kernel_size=1, input_shape=(None, None, in_c))
self.pos_code = self.__getPosCode(fm_sz, out_c)
self.softmax = keras.layers.Softmax(axis=2)
self.pos_bias = pos_bias
def __getPosCode(self, fm_sz, out_c):
x = []
for i in range(fm_sz):
x.append([np.sin, np.cos][i % 2](1 / (10000 ** (i // 2 / fm_sz))))
x = tf.convert_to_tensor([x], dtype=tf.float32)
return tf.concat([(x + tf.transpose(x)).unsqueeze(0) for i in range(out_c)], axis=0)
def call(self, x):
q, k, v = self.w_q(x), self.w_k(x), self.w_v(x)
pos_code = tf.concat([self.pos_code.unsqueeze(0) for i in range(x.shape[0])], axis=0)
if self.pos_bias:
att_map = tf.matmul(q, tf.transpose(k, perm=[0, 1, 3, 2])) + pos_code
else:
att_map = tf.matmul(q, tf.transpose(k, perm=[0, 1, 3, 2])) + tf.matmul(q, tf.transpose(pos_code, perm=[0, 1, 3, 2]))
am_shape = att_map.shape
att_map = self.softmax(tf.reshape(att_map, [am_shape[0], am_shape[1], am_shape[2] * am_shape[3]]))
att_map = tf.reshape(att_map, am_shape)
return att_map * v
```
需要注意的是,这里的代码只是一种可能的TensorFlow实现方式,具体还需要根据实际情况进行微调。
阅读全文
相关推荐
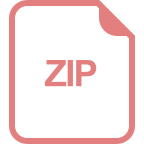
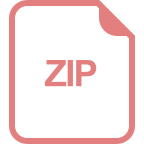
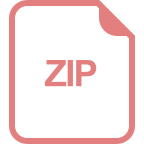














