stm32蓝牙小车代码
时间: 2023-09-09 13:07:18 浏览: 38
以下是基于STM32单片机和HC-05蓝牙模块的小车控制代码,你可以根据自己的需要进行修改:
```c
#include "stm32f10x.h"
#define CMD_FORWARD 'w'
#define CMD_BACKWARD 's'
#define CMD_LEFT 'a'
#define CMD_RIGHT 'd'
#define CMD_STOP 'x'
void delay_ms(uint32_t ms);
void uart_init(uint32_t baudrate);
void uart_send_byte(uint8_t data);
void motor_control(char cmd);
int main(void) {
uart_init(9600);
while (1) {
char cmd = getchar();
motor_control(cmd);
}
}
void delay_ms(uint32_t ms) {
uint32_t i, j;
for (i = 0; i < ms; i++)
for (j = 0; j < 5000; j++);
}
void uart_init(uint32_t baudrate) {
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
// enable clock for GPIOA and USART2
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1, ENABLE);
// configure USART2 TX (PA2) and RX (PA3) as alternate function push-pull
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// configure USART2
USART_InitStruct.USART_BaudRate = baudrate;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_Init(USART1, &USART_InitStruct);
// enable USART2 IRQ in NVIC
NVIC_InitStruct.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_Init(&NVIC_InitStruct);
// enable USART2 RX interrupt
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// enable USART2
USART_Cmd(USART1, ENABLE);
}
void uart_send_byte(uint8_t data) {
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, data);
}
void motor_control(char cmd) {
switch (cmd) {
case CMD_FORWARD:
// code to move the car forward
break;
case CMD_BACKWARD:
// code to move the car backward
break;
case CMD_LEFT:
// code to turn the car left
break;
case CMD_RIGHT:
// code to turn the car right
break;
case CMD_STOP:
// code to stop the car
break;
default:
// invalid command, do nothing
break;
}
}
```
注意,以上代码仅供参考,具体实现需要根据你的硬件和需求进行修改。
相关推荐
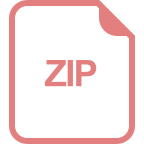














