一个csv文件,算出三行合并的值
时间: 2024-09-11 08:07:17 浏览: 41
CSV(Comma Separated Values)文件是一种常见的文本文件格式,用于存储表格数据,其中每一行代表一行记录,每个字段由逗号分隔。如果你想计算三个连续行的某个字段相加的总和,首先你需要读取这个文件,并逐行处理。
例如,在Python中,你可以使用pandas库来操作CSV文件:
```python
import pandas as pd
# 读取CSV文件
data = pd.read_csv('your_file.csv')
# 假设你想合并前三行的某一列(这里假设为'column_name')
sum_of_first_three_rows = data.iloc[:3]['column_name'].sum()
```
在这个例子中,`iloc[:3]`选择前三个行,然后对指定的'column_name'列求和。记得替换 'your_file.csv' 和 'column_name' 为你实际文件名和需要计算的列名。
如果你直接在命令行操作CSV,可以使用awk或其他文本处理工具,如在Unix/Linux系统中:
```bash
awk -F ',' '{total += $n} END {print total}' your_file.csv > sum.txt
```
这里 `-F ','` 指定分隔符为逗号,`$n` 表示你想要累计的列的索引位置,比如 `3` 如果你想累加第三列。结果会输出到一个新的文件 `sum.txt` 中。
相关问题
pyhton合并三个csv文件为一个
你可以使用 pandas 库来合并三个 CSV 文件。以下是一个示例代码:
```python
import pandas as pd
# 读取三个 CSV 文件
df1 = pd.read_csv('file1.csv')
df2 = pd.read_csv('file2.csv')
df3 = pd.read_csv('file3.csv')
# 合并三个数据框
merged_df = pd.concat([df1, df2, df3])
# 将合并后的数据框保存为新的 CSV 文件
merged_df.to_csv('merged_file.csv', index=False)
```
这个代码假设三个 CSV 文件的结构相同,即它们具有相同的列名和数据类型。如果三个文件的结构不同,你可能需要进行一些数据清洗和转换,以使它们具有相同的结构。
合并多个csv文件除第一行以外的全部内容
可以使用Python的pandas库来合并多个csv文件除第一行以外的全部内容。下面是一个示例代码:
```python
import pandas as pd
import glob
# 获取所有csv文件的文件名
file_names = glob.glob('*.csv')
# 读入第一个csv文件的全部内容
df = pd.read_csv(file_names[0], skiprows=[1])
# 循环遍历其他csv文件,将数据合并到df中
for file in file_names[1:]:
temp_df = pd.read_csv(file, skiprows=[1])
df = pd.concat([df, temp_df])
# 将合并后的数据保存到文件中
df.to_csv('merged.csv', index=False)
```
注意这里的`skiprows=[1]`参数将第一行跳过,不会被合并到结果中。最后合并后的数据将保存到文件`merged.csv`中。
阅读全文
相关推荐
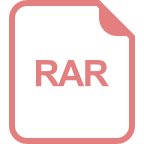
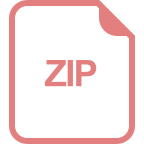
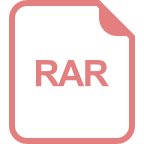













