用C++的opencv写一段代码,要求能够识别红蓝色的圆环,能够标出圆心坐标,并且使用键盘Q键,使得能够红蓝模式切换
时间: 2023-05-26 18:01:30 浏览: 58
以下是使用C++的OpenCV库实现的代码,可以检测红色或蓝色的圆环,识别圆心坐标,并使用Q键在红蓝模式之间切换。
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
bool blue_mode = false; // false表示红色模式,true表示蓝色模式
VideoCapture cap(0);
if (!cap.isOpened()) {
cout << "无法打开相机" << endl;
return -1;
}
namedWindow("circles", WINDOW_NORMAL);
while (true) {
Mat frame, img_hsv, img_red, img_blue;
cap.read(frame);
if (frame.empty()) {
cout << "无法读取帧" << endl;
break;
}
// 将图像转换为HSV颜色空间
cvtColor(frame, img_hsv, COLOR_BGR2HSV);
// 在红色范围内创建二值化图像
inRange(img_hsv, Scalar(0, 70, 50), Scalar(10, 255, 255), img_red);
inRange(img_hsv, Scalar(100, 70, 50), Scalar(124, 255, 255), img_blue);
// 合并两个二值化图像
Mat img_circles;
if (!blue_mode) {
img_circles = img_red;
}
else {
img_circles = img_blue;
}
// 检测圆环
GaussianBlur(img_circles, img_circles, Size(9, 9), 2, 2);
vector<Vec3f> circles;
HoughCircles(img_circles, circles, HOUGH_GRADIENT, 1,
img_circles.rows / 16, 100, 30, 10, 50);
// 绘制圆环和圆心
if (!circles.empty()) {
for (size_t i = 0; i < circles.size(); i++) {
Vec3i c = circles[i];
Point center = Point(c[0], c[1]);
int radius = c[2];
circle(frame, center, radius, Scalar(0, 255, 0), 2);
circle(frame, center, 2, Scalar(0, 0, 255), 3);
}
}
// 显示图像
imshow("circles", frame);
// 键盘事件处理
char key = waitKey(1);
if (key == 'q' || key == 'Q') {
blue_mode = !blue_mode; // 切换红蓝模式
}
else if (key == 27) { // ESC退出程序
break;
}
}
return 0;
}
```
相关推荐
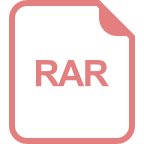














