func randomChain() chan interface{} { // 1 避免丢失数据 nc := make(chan interface{}, 1) count := int64(0) go func() { ticker := time.NewTicker(1 * time.Second) for { select { case <-ticker.C: fmt.Println("count:", count) count = 0 default: nc <- &Data{rand.Int63()} count++ } } }() return nc } 优化
时间: 2024-02-28 20:53:12 浏览: 95
这段代码的问题在于它使用了一个固定大小的缓冲通道,当缓冲通道被填满时,新的数据会被丢弃。这可能会导致数据的丢失,因此需要进行优化。
一种优化方法是使用一个动态大小的缓冲通道。我们可以使用无缓冲通道作为计数器,每当发送一个新的数据时,增加计数器的值。同时,使用一个 for 循环不断从计数器中读取值,并根据计数器的值动态调整缓冲通道的大小。
下面是优化后的代码:
```
func randomChain() chan interface{} {
nc := make(chan interface{})
count := int64(0)
go func() {
ticker := time.NewTicker(1 * time.Second)
for {
select {
case <-ticker.C:
fmt.Println("count:", count)
count = 0
default:
nc <- &Data{rand.Int63()}
count++
// 动态调整缓冲通道大小
if count%100 == 0 {
newSize := len(nc) + 10
if newSize > cap(nc) {
newCh := make(chan interface{}, newSize)
for i := 0; i < len(nc); i++ {
newCh <- <-nc
}
nc = newCh
}
}
}
}
}()
return nc
}
```
这段代码使用一个 for 循环不断从计数器中读取值,并根据计数器的值动态调整缓冲通道的大小。每当计数器的值为 100 的倍数时,我们将缓冲通道的大小增加 10。如果增加后的大小大于缓冲通道的容量,我们会创建一个新的缓冲通道,并将旧的通道中的数据转移到新的通道中。这样就可以避免数据丢失的问题。
阅读全文
相关推荐
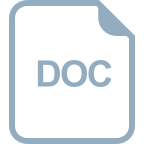
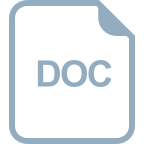
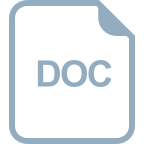








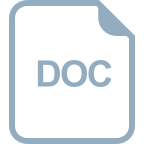
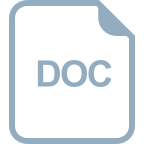
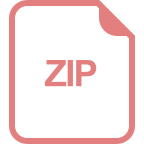
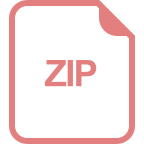
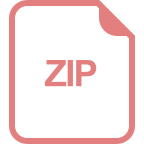
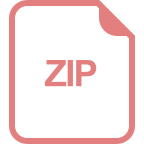
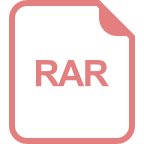