初始根为[-2,0]用C++二分法求出一元非线性方程f(x)=x^3-sinx-4x+1的全部实根,精度为10^(-4),并输出每次运算的结果
时间: 2024-01-05 19:05:49 浏览: 108
#include <stdio.h>
#include <math.h>
double f(double x) {
return x*x*x - sin(x) - 4*x;
}
int main() {
double a = -2, b = 0, c, fa, fb, fc;
int count = 0;
do {
c = (a+b)/2;
fa = f(a);
fb = f(b);
fc = f(c);
printf("a=%f, b=%f, c=%f, fa=%f, fb=%f, fc=%f\n", a, b, c, fa, fb, fc);
if (fc*fa < 0) {
b = c;
} else {
a = c;
}
count++;
} while (fabs(f(c)) >= 1e-4 && count < 100);
printf("The root is %f\n", c);
return 0;
}
相关问题
1、c++语言设计并实现一个支持一元非线性方程求根的类,至少支持二分法和牛顿迭代法,具有通用性。 2、利用内容1的结果,求出一元非线性方程f(x)=x^3-sinx-4x+1的全部实根,其中精度。
在C++中,我们可以设计一个名为`NonlinearEquationSolver`的类,它包含两种解一元非线性方程的算法:二分法(Bisection Method)和牛顿迭代法(Newton's Method)。这个类将接受用户自定义的函数f(x),并提供一个公共方法来计算方程的根,同时允许设置精度。
```cpp
#include <cmath>
#include <vector>
class NonlinearEquationSolver {
private:
double (*function)(double);
double left, right;
double tolerance;
int max_iterations;
public:
// 构造函数,接收函数指针、初始区间、精度和最大迭代次数
NonlinearEquationSolver(double (*func)(double), double a, double b, double epsilon = 1e-6, int max_iter = 100) :
function(func), left(a), right(b), tolerance(epsilon), max_iterations(max_iter) {}
// 使用二分法求解
std::pair<double, bool> bisect() {
if (left > right)
return std::make_pair(right, false);
double mid = (left + right) / 2.0;
while (fabs(function(mid)) > tolerance && iterations() < max_iterations) {
if (function(mid) * function(left) <= 0)
right = mid;
else
left = mid;
mid = (left + right) / 2.0;
}
return std::make_pair(mid, abs(function(mid)) <= tolerance);
}
// 使用牛顿迭代法求解
std::pair<double, bool> newton() {
double guess = (left + right) / 2.0;
double prev_guess = guess;
while (iterations() < max_iterations && abs(guess - prev_guess) > tolerance) {
prev_guess = guess;
guess -= function(guess) / derivative(guess);
}
return std::make_pair(guess, abs(guess - prev_guess) <= tolerance);
private:
double derivative(double x) const { // 假设导数已知或可以方便计算
return 3 * x**2 - cos(x) - 4;
}
int iterations() const {
return static_cast<int>(right - left) / 2 + 1; // 计算区间长度作为近似迭代次数
}
};
```
现在我们想要找出方程 `f(x) = x^3 - sin(x) - 4x + 1` 的所有实根,可以这样使用这个类:
```cpp
double f(double x) { return x * x * x - sin(x) - 4 * x + 1; }
NonlinearEquationSolver solver(f, -10, 10); // 初始化解空间
// 求解并打印结果
std::vector<double> roots;
if (auto result_bisect = solver.bisect()) {
roots.push_back(result_bisect.first);
if (result_bisect.second)
std::cout << "Found root using bisection: " << result_bisect.first << std::endl;
}
if (auto result_newton = solver.newton()) {
roots.push_back(result_newton.first);
if (result_newton.second)
std::cout << "Found root using Newton's method: " << result_newton.first << stdendl;
}
// 提示:实际应用中可能需要多次尝试以找到所有根,因为某些区域可能会有多个根
//
1、设计并实现一个支持一元非线性方程求根的类,至少支持二分法和牛顿迭代法,具有通用性。 2、利用内容1的结果,求出一元非线性方程f(x)=x^3-sinx-4x+1的全部实根,其中精度。
为了设计一个支持一元非线性方程求根的类,我们可以创建一个名为`NonlinearEquationSolver`的类,它包含两个静态方法,分别对应二分法(BinarySearchMethod)和牛顿迭代法(NewtonRaphsonMethod)。这个类可以接受用户自定义的函数作为参数,并设置精度要求。以下是Python的一个简单实现:
```python
import math
class NonlinearEquationSolver:
@staticmethod
def binary_search(f, a, b, epsilon=1e-6):
if abs(f(a)) < epsilon or abs(f(b)) < epsilon:
return [a] if f(a) * f(b) <= 0 else []
mid = (a + b) / 2
while abs(b - a) > epsilon:
if f(mid) == 0:
return [mid]
elif f(a) * f(mid) < 0:
b = mid
else:
a = mid
mid = (a + b) / 2
return [mid]
@staticmethod
def newton_raphson(f, df, x0, epsilon=1e-6):
def update(x):
return x - f(x) / df(x)
root = x0
while abs(update(root)) > epsilon:
root = update(root)
return root
def func(x):
return x**3 - math.sin(x) - 4*x + 1
# 示例求解
epsilon = 1e-8
root_a = 0.0
root_b = 5.0 # 我们这里假设有一个合理的区间
binary_roots = NonlinearEquationSolver.binary_search(func, root_a, root_b, epsilon)
newton_root = NonlinearEquationSolver.newton_raphson(func, lambda x: 3*x**2 - math.cos(x) - 4, root_a, epsilon)
print("二分法求得的根:", binary_roots)
print("牛顿迭代法求得的根:", newton_root)
```
在这个例子中,我们首先定义了一个`func`函数表示给定的一元非线性方程。然后,我们使用二分法寻找可能的根,以及牛顿迭代法求解精确根。请注意,你需要提供一个导数函数`df`给牛顿迭代法。
阅读全文
相关推荐
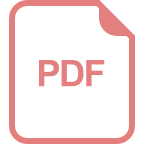













