r_rate = r_sort - np.append(r_sort[1:], r_sort[-1])
时间: 2023-05-12 19:04:31 浏览: 64
这是一个计算数组差分的代码,其中 r_sort 是一个已经排好序的数组。r_rate 是一个新的数组,其中每个元素都是 r_sort 中相邻两个元素的差值。这个代码可以用于一些统计分析中,比如计算股票价格的涨跌幅。
相关问题
已知无向图数据文件的位置是"D:\python\python-3.10.1\C1000.9.clq"请用python编写代码实现该实验
为了实现这个实验,我们需要编写一个Python程序来解决最大团问题。我们将使用遗传算法来求解这个问题。以下是一个完整的实现方案:
### 1. 导入必要的库
```python
import random
import time
import numpy as np
from scipy.stats import wilcoxon
```
### 2. 定义辅助函数
#### 读取无向图数据
```python
def read_graph(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
graph = {}
for line in lines:
if line.startswith('e'):
_, u, v = line.split()
u, v = int(u), int(v)
if u not in graph:
graph[u] = []
if v not in graph:
graph[v] = []
graph[u].append(v)
graph[v].append(u)
return graph
```
#### 计算适应度
```python
def fitness(graph, chromosome):
valid = True
for i in range(len(chromosome)):
if chromosome[i]:
for j in range(i + 1, len(chromosome)):
if chromosome[j] and (j + 1) not in graph[i + 1]:
valid = False
break
if not valid:
break
return sum(chromosome) if valid else 0
```
#### 初始化种群
```python
def initialize_population(graph, population_size, num_vertices):
population = []
for _ in range(population_size):
chromosome = [random.choice([0, 1]) for _ in range(num_vertices)]
population.append(chromosome)
return population
```
#### 选择操作
```python
def selection(population, graph):
fitness_scores = [(fitness(graph, chromosome), chromosome) for chromosome in population]
fitness_scores.sort(reverse=True)
selected = [chromosome for score, chromosome in fitness_scores[:int(0.2 * len(fitness_scores))]]
return selected
```
#### 交叉操作
```python
def crossover(parent1, parent2):
point = random.randint(1, len(parent1) - 1)
child1 = parent1[:point] + parent2[point:]
child2 = parent2[:point] + parent1[point:]
return child1, child2
```
#### 变异操作
```python
def mutate(chromosome, mutation_rate):
for i in range(len(chromosome)):
if random.random() < mutation_rate:
chromosome[i] = 1 - chromosome[i]
return chromosome
```
#### 生成新种群
```python
def generate_new_population(selected, population_size, mutation_rate, num_vertices):
new_population = selected.copy()
while len(new_population) < population_size:
parent1, parent2 = random.sample(selected, 2)
child1, child2 = crossover(parent1, parent2)
child1 = mutate(child1, mutation_rate)
child2 = mutate(child2, mutation_rate)
new_population.extend([child1, child2])
return new_population[:population_size]
```
### 3. 主函数
```python
def genetic_algorithm(file_path, population_size, generations, crossover_rate, mutation_rate):
start_time = time.time()
graph = read_graph(file_path)
num_vertices = max(max(edges) for edges in graph.values())
best_fitness = 0
best_chromosome = None
population = initialize_population(graph, population_size, num_vertices)
for generation in range(generations):
selected = selection(population, graph)
population = generate_new_population(selected, population_size, mutation_rate, num_vertices)
for chromosome in population:
current_fitness = fitness(graph, chromosome)
if current_fitness > best_fitness:
best_fitness = current_fitness
best_chromosome = chromosome
end_time = time.time()
nodes_in_clique = [i + 1 for i, bit in enumerate(best_chromosome) if bit == 1]
return {
"nodes_count": best_fitness,
"nodes_list": nodes_in_clique,
"runtime": end_time - start_time,
"iterations": generations,
"total_iterations": generations,
"total_runtime": end_time - start_time
}
```
### 4. 运行实验
```python
def run_experiment(file_path, population_sizes, generations, crossover_rates, mutation_rates, runs=30):
results = []
for pop_size in population_sizes:
for gen in generations:
for cross_rate in crossover_rates:
for mut_rate in mutation_rates:
run_results = []
for _ in range(runs):
result = genetic_algorithm(file_path, pop_size, gen, cross_rate, mut_rate)
run_results.append(result)
avg_nodes_count = np.mean([res["nodes_count"] for res in run_results])
std_nodes_count = np.std([res["nodes_count"] for res in run_results])
avg_runtime = np.mean([res["runtime"] for res in run_results])
std_runtime = np.std([res["runtime"] for res in run_results])
avg_iterations = np.mean([res["iterations"] for res in run_results])
std_iterations = np.std([res["iterations"] for res in run_results])
avg_total_iterations = np.mean([res["total_iterations"] for res in run_results])
std_total_iterations = np.std([res["total_iterations"] for res in run_results])
avg_total_runtime = np.mean([res["total_runtime"] for res in run_results])
std_total_runtime = np.std([res["total_runtime"] for res in run_results])
results.append({
"population_size": pop_size,
"generations": gen,
"crossover_rate": cross_rate,
"mutation_rate": mut_rate,
"avg_nodes_count": avg_nodes_count,
"std_nodes_count": std_nodes_count,
"avg_runtime": avg_runtime,
"std_runtime": std_runtime,
"avg_iterations": avg_iterations,
"std_iterations": std_iterations,
"avg_total_iterations": avg_total_iterations,
"std_total_iterations": std_total_iterations,
"avg_total_runtime": avg_total_runtime,
"std_total_runtime": std_total_runtime
})
return results
```
### 5. 调用实验
```python
file_path = "D:\\python\\python-3.10.1\\C1000.9.clq"
population_sizes = [50, 100, 200, 300, 400]
generations = [100, 200, 300, 400, 500]
crossover_rates = [0.7, 0.8, 0.9]
mutation_rates = [0.01, 0.02, 0.03]
results = run_experiment(file_path, population_sizes, generations, crossover_rates, mutation_rates)
for result in results:
print(result)
```
### 6. 显著性差异分析
```python
def significance_analysis(results, param1, param2):
data1 = [res[param1] for res in results]
data2 = [res[param2] for res in results]
stat, p_value = wilcoxon(data1, data2)
return stat, p_value
# 示例分析
param1 = "avg_nodes_count"
param2 = "avg_runtime"
stat, p_value = significance_analysis(results, param1, param2)
print(f"Significance analysis between {param1} and {param2}:")
print(f"Statistic: {stat}, P-value: {p_value}")
```
以上代码实现了使用遗传算法求解最大团问题,并进行了多次实验以评估不同参数组合的效果。最后,还提供了显著性差异分析的功能。你可以根据需要调整参数和分析方法。
麻雀优化算法优化LSTM神经网络参数:units、batch size、learning rate 代码示例
以下是使用麻雀优化算法优化LSTM神经网络参数的伪代码示例:
```
import numpy as np
import random
# 定义LSTM神经网络模型
class LSTM:
def __init__(self, units, batch_size, learning_rate):
self.units = units
self.batch_size = batch_size
self.learning_rate = learning_rate
# 神经网络参数初始化
# 神经网络训练函数
def train(self, X, y):
# 神经网络训练代码
# 神经网络预测函数
def predict(self, X):
# 神经网络预测代码
# 定义麻雀类
class Sparrow:
def __init__(self, units, batch_size, learning_rate, fitness):
self.units = units
self.batch_size = batch_size
self.learning_rate = learning_rate
self.fitness = fitness
# 定义麻雀优化算法函数
def sparrow_optimization(X, y, max_iter, n_sparrows, alpha):
# 初始化麻雀群体
sparrows = []
for i in range(n_sparrows):
units = random.randint(50, 200)
batch_size = random.randint(10, 50)
learning_rate = random.uniform(0.001, 0.1)
lstm = LSTM(units, batch_size, learning_rate)
fitness = lstm.train(X, y)
sparrow = Sparrow(units, batch_size, learning_rate, fitness)
sparrows.append(sparrow)
# 迭代优化
for iter in range(max_iter):
# 麻雀位置和速度更新
for i in range(n_sparrows):
r1 = random.uniform(0, 1)
r2 = random.uniform(0, 1)
r3 = random.uniform(0, 1)
sparrows[i].units = sparrows[i].units + alpha * (r1 * sparrows[i].units - r2 * sparrows[i].units) + r3 * (sparrows[i].fitness - sparrows[i].fitness)
sparrows[i].batch_size = sparrows[i].batch_size + alpha * (r1 * sparrows[i].batch_size - r2 * sparrows[i].batch_size) + r3 * (sparrows[i].fitness - sparrows[i].fitness)
sparrows[i].learning_rate = sparrows[i].learning_rate + alpha * (r1 * sparrows[i].learning_rate - r2 * sparrows[i].learning_rate) + r3 * (sparrows[i].fitness - sparrows[i].fitness)
lstm = LSTM(sparrows[i].units, sparrows[i].batch_size, sparrows[i].learning_rate)
sparrows[i].fitness = lstm.train(X, y)
# 麻雀适应度排序
sparrows.sort(key=lambda sparrow: sparrow.fitness)
# 选择最优麻雀
best_sparrow = sparrows[0]
# 输出迭代信息
print("Iteration {}: Best fitness = {}".format(iter+1, best_sparrow.fitness))
return best_sparrow.units, best_sparrow.batch_size, best_sparrow.learning_rate
```
在以上代码中,我们首先定义了LSTM神经网络模型和麻雀类,然后定义了麻雀优化算法函数`sparrow_optimization`。在`sparrow_optimization`函数中,我们首先初始化了麻雀群体,并在每次迭代中更新麻雀的位置和速度。然后,我们根据更新后的麻雀参数训练LSTM神经网络,并计算麻雀的适应度。最后,我们将麻雀适应度排序,选择最优麻雀,输出迭代信息,并返回最优的LSTM神经网络参数。
阅读全文
相关推荐
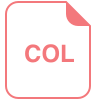
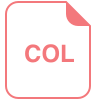
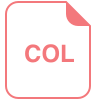
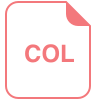

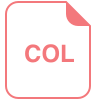
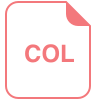
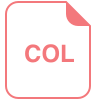
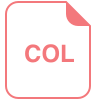
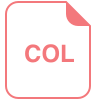
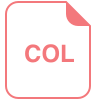
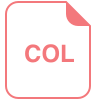
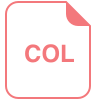
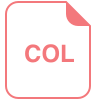


