随机化算法入门指南:揭开算法的神秘面纱
发布时间: 2024-08-24 18:22:48 阅读量: 16 订阅数: 29 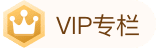
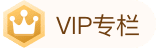
# 1. 随机化算法概述**
随机化算法是一种独特的算法范式,它利用随机性来解决计算问题。与传统算法不同,随机化算法在执行过程中引入随机因素,从而获得更优的性能或解决原本无法解决的问题。
随机化算法的本质在于,它通过引入随机性来打破计算过程中的确定性,从而探索不同的解决方案空间。这种随机性使得算法能够跳出局部最优解,找到更好的全局解,或者在不确定性环境中做出更鲁棒的决策。
# 2. 随机化算法基础
### 2.1 概率论基础
#### 2.1.1 概率分布
概率分布描述了随机变量取值的可能性。常见的概率分布包括:
- **均匀分布:**所有取值具有相等的概率。
- **二项分布:**表示在 n 次独立试验中成功 k 次的概率。
- **正态分布:**又称高斯分布,表示连续随机变量的概率密度函数。
#### 2.1.2 期望值和方差
- **期望值:**随机变量取值的平均值,表示其取值的中心位置。
- **方差:**随机变量取值与期望值之差的平方值的平均值,表示其取值的离散程度。
### 2.2 随机数生成
#### 2.2.1 伪随机数生成器
伪随机数生成器(PRNG)使用确定性算法生成看似随机的数字序列。常见的 PRNG 包括:
- **线性同余生成器 (LCG):**使用线性同余公式生成随机数。
- **梅森旋转算法 (MT):**一种基于梅森素数的 PRNG,具有较长的周期。
#### 2.2.2 真随机数生成
真随机数生成器(TRNG)使用物理现象(如热噪声或量子效应)生成真正的随机数。它们比 PRNG 更安全,但生成速度较慢。
**代码示例:**
```python
import random
# 使用 PRNG 生成随机数
random_number = random.random() # 生成 [0, 1) 之间的随机浮点数
# 使用 TRNG 生成随机数
import os
random_bytes = os.urandom(16) # 生成 16 字节的真随机字节
```
**代码逻辑分析:**
* `random.random()` 函数使用 Mersenne Twister PRNG 生成一个 [0, 1) 之间的浮点数。
* `os.urandom()` 函数使用系统提供的 TRNG 生成指定字节数的真随机字节。
# 3. 随机化算法应用
### 3.1 排序算法
#### 3.1.1 快速排序
快速排序是一种基于分治思想的随机化排序算法,其平均时间复杂度为 O(n log n),最坏时间复杂度为 O(n^2)。
**算法步骤:**
1. 从数组中随机选择一个元素作为枢纽。
2. 将数组划分为两部分:比枢纽小的元素和比枢纽大的元素。
3. 递归地对两部分进行快速排序。
**代码块:**
```python
def quick_sort(arr):
if len(arr) <= 1:
return arr
# 随机选择枢纽
pivot = random.choice(arr)
# 分割数组
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
# 递归排序
return quick_sort(left) + middle + quick_sort(right)
```
**逻辑分析:**
* `random.choice(arr)` 随机选择一个元素作为枢纽。
* `[x for x in arr if x < pivot]` 过滤出比枢纽小的元素。
* `[x for x in arr if x == pivot]` 过滤出等于枢纽的元素。
* `[x for x in arr if x > pivot]` 过滤出比枢纽大的元素。
* 递归调用 `quick_sort(left)` 和 `quick_sort(right)` 对两部分进行排序。
#### 3.1.2 归并排序
归并排序是一种稳定的排序算法,其时间复杂度为 O(n log n)。
**算法步骤:**
1. 将数组分为两半。
2. 递归地对两半进行归并排序。
3. 合并两个已排序的子数组。
**代码块:**
```python
def merge_sort(arr):
if len(arr) <= 1:
return arr
# 分割数组
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
# 合并两个子数组
return merge(left, right)
def merge(left, right):
i, j = 0, 0
merged = []
while i < len(left) and j < len(right):
if left[i] < right[j]:
merged.append(left[i])
i += 1
else:
merged.append(right[j])
j += 1
while i < len(left):
merged.append(left[i])
i += 1
while j < len(right):
merged.append(right[j])
j += 1
return merge
```
0
0
相关推荐
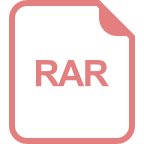
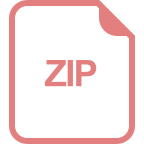
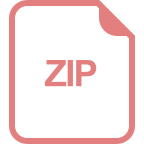