运用C语言编写一个完整的程序,利用顺序表的基本操作完成两个多项式的加法运算。算法思路:用两个顺序表分别存储1元多项式p1和p2,依次将p1和p二对应下标的元素相加,结果存于p1,如果p2中的元素多余p1,将p2中多出的元素全部复制到p1
时间: 2024-12-02 14:21:08 浏览: 28
以下是一个简单的C语言程序,用于计算两个一元多项式的加法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义多项式节点结构
typedef struct Term {
double coefficient; // 系数
int exponent; // 指数
} Term;
// 创建顺序表的函数
Term* create_list(double coefficients[], int degree) {
Term* head = (Term*)malloc(sizeof(Term));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->coefficient = coefficients[0];
head->exponent = 0;
for (int i = 1; i <= degree; i++) {
Term* newNode = (Term*)malloc(sizeof(Term));
newNode->coefficient = coefficients[i];
newNode->exponent = i;
newNode->next = head->next;
head->next = newNode;
}
return head;
}
// 合并多项式函数
Term* add_polynomials(Term* p1, Term* p2) {
Term* tail = p1;
while (p2 != NULL) {
// 如果p1还有空间,直接添加
if (tail->next != NULL) {
tail->next->coefficient += p2->coefficient;
tail = tail->next;
}
// 否则将p2剩余部分移到p1
else {
tail->next = p2;
while (p2->next != NULL) {
tail = tail->next;
p2 = p2->next;
}
}
p2 = p2->next;
}
return p1;
}
// 打印多项式函数
void print_polynomial(Term* head) {
while (head != NULL) {
printf("%.2f*x^%d + ", head->coefficient, head->exponent);
head = head->next;
}
printf("0\n");
}
int main() {
// 示例多项式系数
double p1_coefficients[] = {1, 2, 3}; // 1x^0 + 2x^1 + 3x^2
double p2_coefficients[] = {4, 5}; // 4x^0 + 5x^1
int p1_degree = sizeof(p1_coefficients) / sizeof(p1_coefficients[0]) - 1;
int p2_degree = sizeof(p2_coefficients) / sizeof(p2_coefficients[0]) - 1;
Term* p1 = create_list(p1_coefficients, p1_degree);
Term* p2 = create_list(p2_coefficients, p2_degree);
// 加法操作
Term* result = add_polynomials(p1, p2);
// 打印结果多项式
printf("Sum of polynomials:\n");
print_polynomial(result);
// 清理内存
while (result != NULL) {
Term* temp = result;
result = result->next;
free(temp);
}
return 0;
}
```
这个程序首先定义了多项式节点结构,并实现了创建列表、合并多项式和打印多项式的功能。在`main`函数中,我们创建了两个一元多项式,然后通过`add_polynomials`函数进行加法运算,并打印出结果。
注意:为了简化示例,这里没有考虑指数相加可能导致结果超出预期的问题,实际应用中应根据实际情况调整。
阅读全文
相关推荐
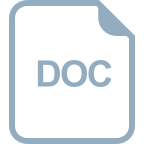
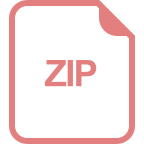
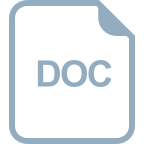
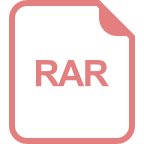
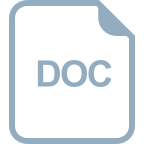
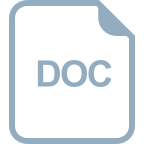
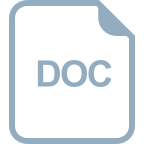
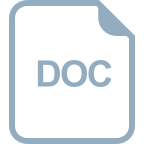
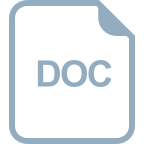
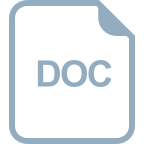
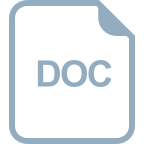
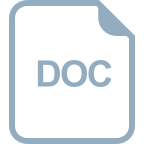



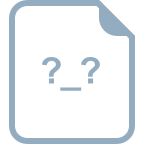
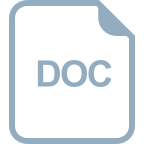
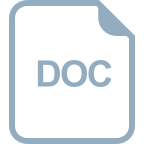
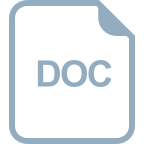