int t_x, t_y; // 按下的坐标 while (1) { // 2.读取触摸屏信息 struct input_event xy; read(fd, &xy, sizeof(xy)); // 判断是否为触摸屏的X 轴事件 if (xy.type == EV_ABS && xy.code == ABS_X) { // 转换坐标 x = xy.value * 800 / 1024; *gx = x; // 输出X轴的坐标值 printf("x=%d\n", x); } // 判断是否为触摸屏的X 轴事件 if (xy.type == EV_ABS && xy.code == ABS_Y) { // 转换坐标 y = xy.value * 480 / 600; *gy = y; // 输出X轴的坐标值 printf("y=%d\n", y); } // 获取按钮是否按下的状态 if (xy.type == EV_KEY && xy.code == BTN_TOUCH) { printf("touch=%d\n", xy.value); if (xy.value == 1) // 按下 { // 保存按下的坐标 t_x = x; t_y = y; } if (xy.value == 0) // 松开 { break; // 跳出循环 } } }
时间: 2024-02-10 10:08:47 浏览: 190
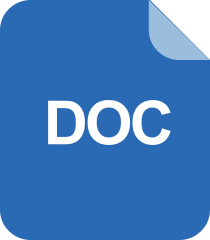
如何确定触摸屏的坐标

这是一段 C 代码,可能是在 Linux 嵌入式系统上针对触摸屏进行的编程。通过读取触摸屏事件,将触摸屏坐标转换成屏幕坐标,并保存按下的坐标和松开的坐标。其中 EV_ABS 和 ABS_X 表示 X 轴方向上的事件,EV_ABS 和 ABS_Y 表示 Y 轴方向上的事件,xy.value 表示事件的值。如果检测到按钮按下和松开的状态,就记录相应的坐标,并跳出循环。这段代码可能被用于开发嵌入式系统上的触摸屏应用程序。你还有其他问题吗?
阅读全文
相关推荐
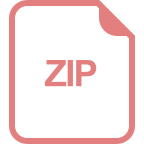
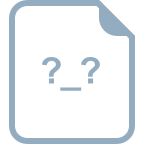
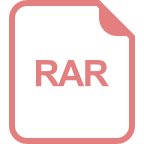
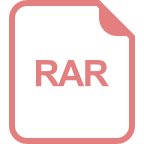













