io模拟iic读TMP102温度
时间: 2023-07-05 09:08:20 浏览: 95
以下是基于STM32F4系列单片机的IO模拟IIC读取TMP102温度的示例代码:
```c
#include "stm32f4xx.h"
#define SDA_PIN GPIO_Pin_9
#define SDA_GPIO_PORT GPIOB
#define SDA_GPIO_CLK RCC_AHB1Periph_GPIOB
#define SCL_PIN GPIO_Pin_8
#define SCL_GPIO_PORT GPIOB
#define SCL_GPIO_CLK RCC_AHB1Periph_GPIOB
#define I2C_SPEED 100000
void IIC_Delay(void) {
uint16_t i = 7 * (SystemCoreClock / 10000000);
while(i--);
}
void IIC_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(SDA_GPIO_CLK | SCL_GPIO_CLK, ENABLE);
GPIO_InitStructure.GPIO_Pin = SDA_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(SDA_GPIO_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SCL_PIN;
GPIO_Init(SCL_GPIO_PORT, &GPIO_InitStructure);
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
}
void IIC_Start(void) {
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
GPIO_ResetBits(SDA_GPIO_PORT, SDA_PIN);
IIC_Delay();
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
}
void IIC_Stop(void) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
GPIO_ResetBits(SDA_GPIO_PORT, SDA_PIN);
IIC_Delay();
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
}
uint8_t IIC_WaitAck(void) {
uint16_t i = 50000;
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
IIC_Delay();
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
while(GPIO_ReadInputDataBit(SDA_GPIO_PORT, SDA_PIN)) {
if(!(i--)) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
return 1;
}
}
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
return 0;
}
void IIC_Ack(void) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
GPIO_ResetBits(SDA_GPIO_PORT, SDA_PIN);
IIC_Delay();
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
}
void IIC_NAck(void) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
IIC_Delay();
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
}
void IIC_WriteByte(uint8_t byte) {
uint8_t i = 8;
while(i--) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
if(byte & 0x80)
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
else
GPIO_ResetBits(SDA_GPIO_PORT, SDA_PIN);
byte <<= 1;
IIC_Delay();
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
}
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
}
uint8_t IIC_ReadByte(uint8_t ack) {
uint8_t i = 8, byte = 0;
GPIO_SetBits(SDA_GPIO_PORT, SDA_PIN);
while(i--) {
GPIO_ResetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
byte <<= 1;
if(GPIO_ReadInputDataBit(SDA_GPIO_PORT, SDA_PIN))
byte |= 0x01;
GPIO_SetBits(SCL_GPIO_PORT, SCL_PIN);
IIC_Delay();
}
if(ack)
IIC_Ack();
else
IIC_NAck();
return byte;
}
void TMP102_Init(void) {
IIC_Start();
IIC_WriteByte(0x90);
IIC_WaitAck();
IIC_WriteByte(0x01);
IIC_WaitAck();
IIC_Stop();
}
float TMP102_ReadTemp(void) {
uint16_t temp;
float t;
IIC_Start();
IIC_WriteByte(0x90);
IIC_WaitAck();
IIC_WriteByte(0x00);
IIC_WaitAck();
IIC_Start();
IIC_WriteByte(0x91);
IIC_WaitAck();
temp = (IIC_ReadByte(1) << 8) | IIC_ReadByte(0);
IIC_Stop();
t = temp * 0.0625;
return t;
}
int main(void) {
float temperature;
RCC_DeInit();
SystemInit();
IIC_Init();
TMP102_Init();
while(1) {
temperature = TMP102_ReadTemp();
// 处理温度数据
}
}
```
在本例中,使用GPIO模拟IIC总线,通过模拟SCL和SDA信号来实现与TMP102的通信。首先初始化GPIO口,然后定义IIC总线的各种操作函数。在主函数中初始化TMP102,并循环读取温度数据。需要注意的是,TMP102的温度数据为16位有符号数,需要经过转换才能得到实际温度值。
相关推荐
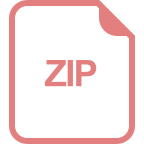














