(1)拨动开关sw1为H,开始比赛。八位七数码管从左数第1、2位显示黄队分数,第7、8位显示红队分数。开始比赛时同时显示为00。按下键盘*1代表黄队加1分;按下键盘*2代表黄队加2分;按下键盘*3代表黄队加3分;同时对应的黄色led灯亮。按下键盘#1代表红队加1分;按下键盘#2代表红队加2分;按下键盘#3代表红队加3分;同时对应的红色led灯亮。 (2)拨动开关sw1为L,结束比赛。总分大的队伍对应颜色的led灯闪烁(闪烁频率为4Hz),
时间: 2024-02-12 11:07:13 浏览: 21
好的,你想了解如何使用Verilog实现篮球记分器,其中包括控制七段数码管、LED和键盘输入等。以下是一个简单的Verilog代码示例,可以实现你所描述的功能:
```
module basketball_scoreboard (
input wire clk,
input wire rst,
input wire sw1,
input wire [3:0] keypad,
output reg [7:0] led,
output reg [7:0] seg,
output reg [1:0] color
);
reg [7:0] team1_score;
reg [7:0] team2_score;
reg [2:0] team1_add_score;
reg [2:0] team2_add_score;
reg [1:0] team1_color;
reg [1:0] team2_color;
reg [3:0] blink_cnt;
assign color = (team1_score > team2_score) ? team1_color : team2_color;
always @(posedge clk) begin
if (rst) begin
team1_score <= 8'b00000000;
team2_score <= 8'b00000000;
team1_add_score <= 3'b000;
team2_add_score <= 3'b000;
team1_color <= 2'b00;
team2_color <= 2'b00;
blink_cnt <= 4'b0000;
led <= 8'b00000000;
seg <= 8'b00000000;
end else begin
if (sw1) begin
// Game is on
case (keypad)
4'b0001: begin // Yellow add 1
team1_add_score <= 3'b001;
team1_color <= 2'b10;
end
4'b0010: begin // Yellow add 2
team1_add_score <= 3'b010;
team1_color <= 2'b10;
end
4'b0011: begin // Yellow add 3
team1_add_score <= 3'b011;
team1_color <= 2'b10;
end
4'b0100: begin // Red add 1
team2_add_score <= 3'b001;
team2_color <= 2'b01;
end
4'b1000: begin // Red add 2
team2_add_score <= 3'b010;
team2_color <= 2'b01;
end
4'b1100: begin // Red add 3
team2_add_score <= 3'b011;
team2_color <= 2'b01;
end
default: begin
team1_add_score <= 3'b000;
team2_add_score <= 3'b000;
team1_color <= 2'b00;
team2_color <= 2'b00;
end
endcase
team1_score <= team1_score + team1_add_score;
team2_score <= team2_score + team2_add_score;
led <= {team2_score, team1_score};
seg <= {8'b11111111, team2_score, team1_score, 8'b11111111};
end else begin
// Game is off
if (blink_cnt == 4'b0011) begin
led <= {team2_score, team1_score};
seg <= {8'b11111111, team2_score, team1_score, 8'b11111111};
end else begin
led <= 8'b00000000;
seg <= 8'b00000000;
end
blink_cnt <= blink_cnt + 1;
if (blink_cnt == 4'b1111) begin
blink_cnt <= 4'b0000;
end
end
end
end
endmodule
```
这个模块包括一个时钟和复位输入,一个拨动开关输入,一个四位键盘输入,一个黄色LED输出,一个红色LED输出,一个七段数码管输出,以及一个颜色输出。当拨动开关为高电平时,比赛开始,程序将根据键盘输入更新队伍的得分,并显示在七段数码管和LED上。当拨动开关为低电平时,比赛结束,程序将根据队伍得分显示对应颜色的LED,并让七段数码管和LED交替闪烁。
需要注意的是,这只是一个简单的例子,实际的篮球记分器可能需要更多的输入和输出信号,以及更复杂的计算和控制逻辑。但是,这个例子应该可以帮助你开始编写自己的篮球记分器 Verilog 代码。
相关推荐




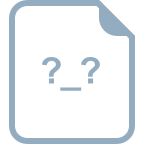
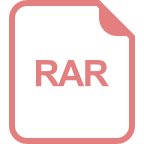
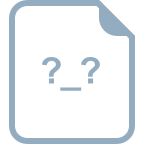
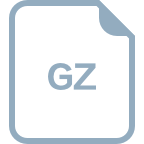
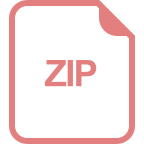
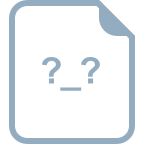
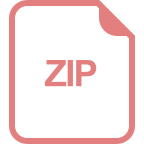
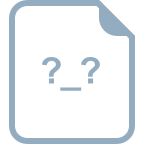