self attention与unet
时间: 2023-07-14 13:05:03 浏览: 65
self attention和UNet是两个不同的概念和模型。
Self-attention是一种注意力机制,用于处理序列数据中的依赖关系。它可以通过计算序列中每个元素与其他元素的关联程度,来为每个元素分配一个权重。这些权重可以用于加权求和,从而获取每个元素的表示。Self-attention在自然语言处理领域非常流行,并被广泛应用于Transformer模型中。
UNet是一种用于图像分割的卷积神经网络结构。它由编码器和解码器两部分组成。编码器部分通过卷积和池化操作逐渐提取图像特征,并进行下采样。解码器部分通过上采样和卷积操作将特征映射恢复到原始图像大小,并进行特征融合。UNet的特点是具有跳跃连接,可以帮助解决分割任务中的细节保留和信息传递问题。
总结起来,self-attention是一种注意力机制,用于处理序列数据中的依赖关系;而UNet是一种用于图像分割的神经网络结构。它们在不同的领域中有着不同的应用和作用。
相关问题
写一个基于self attention 的 unet模型程序
以下是一个基于self attention的unet模型的程序:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class SelfAttentionBlock(nn.Module):
def __init__(self, in_channels):
super(SelfAttentionBlock, self).__init__()
self.query_conv = nn.Conv2d(in_channels, in_channels // 8, kernel_size=1)
self.key_conv = nn.Conv2d(in_channels, in_channels // 8, kernel_size=1)
self.value_conv = nn.Conv2d(in_channels, in_channels, kernel_size=1)
self.gamma = nn.Parameter(torch.zeros(1))
def forward(self, x):
batch_size, C, H, W = x.size()
proj_query = self.query_conv(x).view(batch_size, -1, H * W).permute(0, 2, 1)
proj_key = self.key_conv(x).view(batch_size, -1, H * W)
energy = torch.bmm(proj_query, proj_key)
attention = F.softmax(energy, dim=-1)
proj_value = self.value_conv(x).view(batch_size, -1, H * W)
out = torch.bmm(proj_value, attention.permute(0, 2, 1))
out = out.view(batch_size, C, H, W)
out = self.gamma * out + x
return out
class DoubleConv(nn.Module):
def __init__(self, in_channels, out_channels):
super(DoubleConv, self).__init__()
self.conv = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True),
nn.Conv2d(out_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
def forward(self, x):
x = self.conv(x)
return x
class Up(nn.Module):
def __init__(self, in_channels, out_channels, bilinear=True):
super(Up, self).__init__()
if bilinear:
self.up = nn.Upsample(scale_factor=2, mode='bilinear', align_corners=True)
else:
self.up = nn.ConvTranspose2d(in_channels // 2, in_channels // 2, kernel_size=2, stride=2)
self.conv = DoubleConv(in_channels, out_channels)
def forward(self, x1, x2):
x1 = self.up(x1)
diffY = x2.size()[2] - x1.size()[2]
diffX = x2.size()[3] - x1.size()[3]
x1 = F.pad(x1, [diffX // 2, diffX - diffX // 2,
diffY // 2, diffY - diffY // 2])
x = torch.cat([x2, x1], dim=1)
x = self.conv(x)
return x
class UNet(nn.Module):
def __init__(self, n_channels, n_classes, bilinear=True):
super(UNet, self).__init__()
self.n_channels = n_channels
self.n_classes = n_classes
self.bilinear = bilinear
self.inc = DoubleConv(n_channels, 64)
self.down1 = DoubleConv(64, 128)
self.down2 = DoubleConv(128, 256)
self.down3 = DoubleConv(256, 512)
self.down4 = DoubleConv(512, 1024)
self.up1 = Up(1024, 512, bilinear)
self.up2 = Up(512, 256, bilinear)
self.up3 = Up(256, 128, bilinear)
self.up4 = Up(128, 64, bilinear)
self.outc = nn.Conv2d(64, n_classes, kernel_size=1)
self.sa1 = SelfAttentionBlock(64)
self.sa2 = SelfAttentionBlock(128)
self.sa3 = SelfAttentionBlock(256)
self.sa4 = SelfAttentionBlock(512)
def forward(self, x):
x1 = self.inc(x)
x2 = self.down1(x1)
x2 = self.sa1(x2)
x3 = self.down2(x2)
x3 = self.sa2(x3)
x4 = self.down3(x3)
x4 = self.sa3(x4)
x5 = self.down4(x4)
x5 = self.sa4(x5)
x = self.up1(x5, x4)
x = self.up2(x, x3)
x = self.up3(x, x2)
x = self.up4(x, x1)
x = self.outc(x)
return x
```
希望这个程序能够帮助你!
请写出使用基于pytorch的attention-Unet模型分割乳腺超声图像的代码
我可以提供一个参考,你可以参考以下代码:import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variableclass Attention_Unet(nn.Module):
def __init__(self, in_channels=1, out_channels=1, init_features=32):
super(Attention_Unet, self).__init__()
#Encoder
self.conv1 = nn.Sequential(nn.Conv2d(in_channels, init_features, 3, padding=1),
nn.BatchNorm2d(init_features),
nn.ReLU(inplace=True))
self.conv2 = nn.Sequential(nn.Conv2d(init_features, init_features, 3, padding=1),
nn.BatchNorm2d(init_features),
nn.ReLU(inplace=True))
self.maxpool = nn.MaxPool2d(2, 2)
self.conv3 = nn.Sequential(nn.Conv2d(init_features, init_features*2, 3, padding=1),
nn.BatchNorm2d(init_features*2),
nn.ReLU(inplace=True))
self.conv4 = nn.Sequential(nn.Conv2d(init_features*2, init_features*2, 3, padding=1),
nn.BatchNorm2d(init_features*2),
nn.ReLU(inplace=True))
self.conv5 = nn.Sequential(nn.Conv2d(init_features*2, init_features*4, 3, padding=1),
nn.BatchNorm2d(init_features*4),
nn.ReLU(inplace=True))
self.conv6 = nn.Sequential(nn.Conv2d(init_features*4, init_features*4, 3, padding=1),
nn.BatchNorm2d(init_features*4),
nn.ReLU(inplace=True))
self.conv7 = nn.Sequential(nn.Conv2d(init_features*4, init_features*8, 3, padding=1),
nn.BatchNorm2d(init_features*8),
nn.ReLU(inplace=True))
self.conv8 = nn.Sequential(nn.Conv2d(init_features*8, init_features*8, 3, padding=1),
nn.BatchNorm2d(init_features*8),
nn.ReLU(inplace=True)) #Decoder
self.upconv1 = nn.ConvTranspose2d(init_features*8, init_features*4, 2, stride=2)
self.conv9 = nn.Sequential(nn.Conv2d(init_features*12, init_features*4, 3, padding=1),
nn.BatchNorm2d(init_features*4),
nn.ReLU(inplace=True))
self.conv10 = nn.Sequential(nn.Conv2d(init_features*4, init_features*4, 3, padding=1),
nn.BatchNorm2d(init_features*4),
nn.ReLU(inplace=True))
self.upconv2 = nn.ConvTranspose2d(init_features*4, init_features*2, 2, stride=2)
self.conv11 = nn.Sequential(nn.Conv2d(init_features*6, init_features*2, 3, padding=1),
nn.BatchNorm2d(init_features*2),
nn.ReLU(inplace=True))
self.conv12 = nn.Sequential(nn.Conv2d(init_features*2, init_features*2, 3, padding=1),
nn.BatchNorm2d(init_features*2),
nn.ReLU(inplace=True))
self.upconv3 = nn.ConvTranspose2d(init_features*2, init_features, 2, stride=2)
self.conv13 = nn.Sequential(nn.Conv2d(init_features*3, init_features, 3, padding=1),
nn.BatchNorm2d(init_features),
nn.ReLU(inplace=True))
self.conv14 = nn.Sequential(nn.Conv2d(init_features, init_features, 3, padding=1),
nn.BatchNorm2d(init_features),
nn.ReLU(inplace=True))
self.conv15 = nn.Conv2d(init_features, out_channels, 1) def forward(self, x):
# Encoder
x1 = self.conv1(x)
x2 = self.conv2(x1)
x3 = self.maxpool(x2)
x4 = self.conv3(x3)
x5 = self.conv4(x4)
x6 = self.maxpool(x5)
x7 = self.conv5(x6)
x8 = self.conv6(x7)
x9 = self.maxpool(x8)
x10 = self.conv7(x9)
x11 = self.conv8(x10) # Decoder
x12 = self.upconv1(x11)
x12 = torch.cat((x12, x8), dim=1) # concat along channel axis
x13 = self.conv9(x12)
x14 = self.conv10(x13)
x15 = self.upconv2(x14)
x15 = torch.cat((x15, x5), dim=1)
x16 = self.conv11(x15)
x17 = self.conv12(x16)
x18 = self.upconv3(x17)
x18 = torch.cat((x18, x2), dim=1)
x19 = self.conv13(x18)
x20 = self.conv14(x19)
x21 = self.conv15(x20) return x21
相关推荐
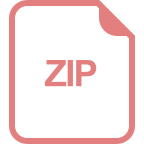
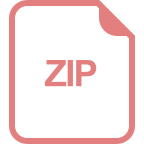
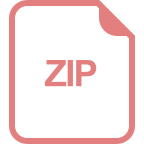













