帮忙写一个stm32中rtc通过备份寄存器校准rtc的完整代码
时间: 2023-12-14 21:37:08 浏览: 28
以下是一个基于STM32的RTC校准代码示例,使用了备份寄存器:
```c
#include "stm32f4xx.h"
#define RTC_BKP_DR0 ((uint32_t)0x0000AABB)
void RTC_Config(void);
ErrorStatus RTC_LSE_Configuration(void);
void RTC_TimeRegulate(uint32_t TimeVar);
void RTC_CalibOutputCmd(FunctionalState NewState);
void RTC_EnterConfigMode(void);
void RTC_ExitConfigMode(void);
int main(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR, ENABLE);
PWR_BackupAccessCmd(ENABLE);
RCC_LSEConfig(RCC_LSE_ON);
while (RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET);
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE);
RCC_RTCCLKCmd(ENABLE);
RTC_Config();
// 从备份寄存器读取校准值
uint32_t bkp_value = RTC_ReadBackupRegister(RTC_BKP_DR0);
if (bkp_value != 0xFFFFFFFF) {
RTC_EnterConfigMode();
RTC_SetCalibrationValue(bkp_value);
RTC_ExitConfigMode();
}
// 获取当前时间
RTC_TimeTypeDef RTC_TimeStructure;
RTC_GetTime(RTC_Format_BIN, &RTC_TimeStructure);
// 校准RTC
RTC_TimeRegulate(RTC_TimeStructure.Time + 10); // 假设误差为10秒
// 将校准值写入备份寄存器
RTC_EnterConfigMode();
RTC_WriteBackupRegister(RTC_BKP_DR0, RTC_GetCalibrationValue());
RTC_ExitConfigMode();
while(1);
}
void RTC_Config(void)
{
RTC_InitTypeDef RTC_InitStructure;
RTC_TimeTypeDef RTC_TimeStructure;
RTC_DateTypeDef RTC_DateStructure;
if (RTC_LSE_Configuration() != SUCCESS) {
// LSE 启动失败
while(1);
}
RTC_InitStructure.RTC_AsynchPrediv = 127;
RTC_InitStructure.RTC_SynchPrediv = 255;
RTC_InitStructure.RTC_HourFormat = RTC_HourFormat_24;
RTC_Init(&RTC_InitStructure);
RTC_TimeStructure.RTC_Hours = 0x00;
RTC_TimeStructure.RTC_Minutes = 0x00;
RTC_TimeStructure.RTC_Seconds = 0x00;
RTC_TimeStructure.RTC_H12 = RTC_H12_AM;
RTC_SetTime(RTC_Format_BIN, &RTC_TimeStructure);
RTC_DateStructure.RTC_Year = 0x00;
RTC_DateStructure.RTC_Month = RTC_Month_January;
RTC_DateStructure.RTC_Date = 0x01;
RTC_DateStructure.RTC_WeekDay = RTC_Weekday_Monday;
RTC_SetDate(RTC_Format_BIN, &RTC_DateStructure);
// 校准输出禁止
RTC_CalibOutputCmd(DISABLE);
}
ErrorStatus RTC_LSE_Configuration(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct;
// 配置LSE
RCC_OscInitStruct.RCC_LSE = RCC_LSE_ON;
RCC_OscInitStruct.RCC_LSEDrive = RCC_LSEDrive_Low;
RCC_OscInitStruct.RCC_OscillatorType = RCC_OscillatorType_LSE;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK) {
return ERROR;
}
// 配置RTC时钟源
RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct;
RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PeriphCLK_RTC;
RCC_PeriphCLKInitStruct.RTCClockSelection = RCC_RTCCLKSource_LSE;
if (HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct) != HAL_OK) {
return ERROR;
}
return SUCCESS;
}
void RTC_TimeRegulate(uint32_t TimeVar)
{
int8_t correction = 0;
// 进入RTC配置模式
RTC_EnterConfigMode();
uint32_t calib_value = RTC_GetCalibrationValue();
if (calib_value == 0xFFFFFFFF) {
// 如果校准值未被设置,则默认为0
correction = -128;
} else {
correction = (int8_t)(calib_value & 0xFF);
}
// 计算新的校准值
correction += (int8_t)((TimeVar - RTC_GetCounter()) / 60);
if (correction > 127) {
correction = 127;
} else if (correction < -128) {
correction = -128;
}
// 设置新的校准值
RTC_SetCalibrationValue(correction);
// 退出RTC配置模式
RTC_ExitConfigMode();
}
void RTC_EnterConfigMode(void)
{
RTC_WaitForSynchro();
RTC_WaitForLastTask();
RTC_ITConfig(RTC_IT_SEC, DISABLE);
RTC_WaitForLastTask();
RTC_EnterConfigMode();
}
void RTC_ExitConfigMode(void)
{
RTC_WaitForSynchro();
RTC_WaitForLastTask();
RTC_ITConfig(RTC_IT_SEC, ENABLE);
RTC_WaitForLastTask();
RTC_ExitConfigMode();
}
```
在上面的示例代码中,我们使用了备份寄存器 RTC\_BKP\_DR0 来存储校准值。在程序启动时,我们从备份寄存器中读取校准值,如果备份寄存器中存在值,则将其设置为 RTC 的校准值。校准完成后,我们将新的校准值写入备份寄存器中,以便下一次启动时使用。
注意:以上代码仅供参考,实际应用中需要根据具体的应用场景进行修改。
相关推荐
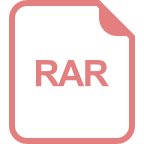














