用qt写一个贪吃蛇游戏,要求按照你的理解就行,并给出实际代码
时间: 2023-09-16 17:05:57 浏览: 89
### 回答1:
使用Qt可以很容易地编写贪吃蛇游戏。下面的代码演示了如何使用Qt来编写一个贪吃蛇游戏:// 包含Qt头文件
#include <QtWidgets>// 定义游戏画面大小
int boardWidth = 20;
int boardHeight = 20;// 初始化游戏
void createBoard()
{
// 创建一个QWidget,用于放置游戏画面
QWidget *window = new QWidget;
window->setFixedSize(boardWidth * 10, boardHeight * 10); // 创建一个QGridLayout,用于放置贪吃蛇和食物
QGridLayout *layout = new QGridLayout;
window->setLayout(layout); // 初始化贪吃蛇的位置
int snakeX = boardWidth / 2;
int snakeY = boardHeight / 2;
layout->addWidget(createSnakeCell(snakeX, snakeY), snakeX, snakeY); // 初始化食物的位置
int foodX = (qrand() % boardWidth);
int foodY = (qrand() % boardHeight);
layout->addWidget(createFoodCell(foodX, foodY), foodX, foodY); window->show();
}// 创建贪吃蛇单元格
QWidget *createSnakeCell(int x, int y)
{
QWidget *cell = new QWidget;
cell->setFixedSize(10, 10);
cell->setStyleSheet("background-color: black;");
return cell;
}// 创建食物单元格
QWidget *createFoodCell(int x, int y)
{
QWidget *cell = new QWidget;
cell->setFixedSize(10, 10);
cell->setStyleSheet("background-color: green;");
return cell;
}int main(int argc, char *argv[])
{
QApplication app(argc, argv); // 创建游戏
createBoard(); return app.exec();
}
### 回答2:
贪吃蛇是一款经典的游戏,在Qt中实现这个游戏可以分为几个主要部分:游戏窗口、蛇的移动、食物与边界碰撞检测以及游戏重启。
首先,创建一个Qt窗口类,用于显示游戏界面。可以使用QWidget或QMainWindow作为基类,根据需求选择。 设置窗口大小、背景颜色和标题。创建一个定时器,用于定时刷新游戏界面。
```c++
class SnakeGame : public QMainWindow
{
Q_OBJECT
public:
SnakeGame(QWidget *parent = nullptr);
~SnakeGame();
private:
void createGame(); // 创建游戏
void moveSnake(); // 蛇的移动
void checkCollision(); // 碰撞检测
void restartGame(); // 重新开始游戏
QTimer* timer; // 定时器
QWidget* gameWidget; // 游戏界面
QGridLayout* gameLayout; // 游戏布局
QVector<QLabel*> snake; // 蛇的身体
QPoint food; // 食物
int direction; // 移动方向(上、下、左、右)
int score; // 分数
};
```
下一步是实现蛇的移动。使用QKeyEvent监听键盘事件,当用户按下方向键时,改变蛇的移动方向。定时器每隔一段时间触发一次moveSnake()方法,根据当前的移动方向更新蛇的坐标,并重新绘制游戏界面。
```c++
void SnakeGame::moveSnake()
{
// 根据移动方向更新蛇头坐标
QPoint newHead = snake[0]->pos();
if (direction == 0)
newHead.setY(newHead.y() - 10);
else if (direction == 1)
newHead.setY(newHead.y() + 10);
else if (direction == 2)
newHead.setX(newHead.x() - 10);
else if (direction == 3)
newHead.setX(newHead.x() + 10);
// 更新蛇身体的坐标
for (int i = snake.size() - 1; i > 0; --i) {
snake[i]->setGeometry(snake[i - 1]->geometry());
}
snake[0]->move(newHead);
// 检测碰撞
checkCollision();
}
```
接下来是碰撞检测。检查蛇是否撞到了边界、自身,或吃到了食物。如果蛇头的坐标与食物的坐标相同,则表示蛇吃到了食物,将食物重新放置在界面上,并增加分数。如果蛇头的坐标与蛇身的坐标重叠,则表示蛇咬到了自己,游戏结束。
```c++
void SnakeGame::checkCollision()
{
QPoint head = snake[0]->pos();
// 检查是否撞到边界
if (head.x() < 0 || head.x() >= gameWidget->width() ||
head.y() < 0 || head.y() >= gameWidget->height()) {
restartGame();
return;
}
// 检查是否撞到自身
for (int i = 1; i < snake.size(); ++i) {
if (head == snake[i]->pos()) {
restartGame();
return;
}
}
// 检查是否吃到食物
if (head == food) {
// 放置新的食物
food.setX(rand() % (gameWidget->width() / 10) * 10);
food.setY(rand() % (gameWidget->height() / 10) * 10);
// 创建新的身体部分
QLabel* newBody = new QLabel(gameWidget);
newBody->setFixedSize(10, 10);
newBody->setStyleSheet("background-color: yellow");
snake.push_back(newBody);
// 更新分数
++score;
}
}
```
最后,实现重启游戏的功能。当游戏结束时,可以重新开始游戏。调用restartGame()方法,清空蛇的身体,重新创建蛇头、食物,分数置零,并重新开始定时器。
```c++
void SnakeGame::restartGame()
{
// 清空蛇的身体
for (int i = 0; i < snake.size(); ++i) {
delete snake[i];
}
snake.clear();
// 创建新的蛇头
QLabel* head = new QLabel(gameWidget);
head->setFixedSize(10, 10);
head->setGeometry(gameWidget->width() / 2 - 5, gameWidget->height() / 2 - 5, 10, 10);
head->setStyleSheet("background-color: green");
snake.push_back(head);
// 创建初始食物
food = QPoint(rand() % (gameWidget->width() / 10) * 10, rand() % (gameWidget->height() / 10) * 10);
// 重置分数和方向
score = 0;
direction = 1;
// 重新开始定时器
timer->start(100);
}
```
以上是基于Qt框架实现贪吃蛇游戏的简单示例。请注意,代码可能需要根据实际情况进行调整和完善。
### 回答3:
贪吃蛇是一款经典的游戏,通过控制一个小蛇在游戏界面上移动,吃到食物来增长身体长度并避免撞到自己或墙壁。我会用Qt编写一个简单的贪吃蛇游戏,并提供一些示例代码。
首先,我们需要创建一个主窗口,并在窗口上绘制游戏场景。使用Qt的QWidget类创建一个GameScene类来实现游戏场景的绘制。
```cpp
#include <QWidget>
#include <QKeyEvent>
class GameScene : public QWidget
{
public:
GameScene(QWidget *parent = nullptr);
~GameScene();
protected:
void paintEvent(QPaintEvent *event);
void keyPressEvent(QKeyEvent *event);
private:
// 蛇的坐标
int snakeX;
int snakeY;
// 食物的坐标
int foodX;
int foodY;
};
GameScene::GameScene(QWidget *parent)
: QWidget(parent),
snakeX(0),
snakeY(0),
foodX(50),
foodY(50)
{
// 设置窗口大小
setFixedSize(400, 400);
// 设置游戏刷新频率
startTimer(1000 / 60);
}
GameScene::~GameScene()
{
}
void GameScene::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
// 绘制蛇
painter.fillRect(snakeX, snakeY, 10, 10, Qt::green);
// 绘制食物
painter.fillRect(foodX, foodY, 10, 10, Qt::red);
}
void GameScene::keyPressEvent(QKeyEvent *event)
{
// 根据按键移动蛇的位置
switch (event->key()) {
case Qt::Key_Up:
snakeY -= 10;
break;
case Qt::Key_Down:
snakeY += 10;
break;
case Qt::Key_Left:
snakeX -= 10;
break;
case Qt::Key_Right:
snakeX += 10;
break;
}
// 更新游戏界面
update();
}
```
接下来,在主窗口中添加一个GameScene对象,并在启动时展示游戏场景。
```cpp
#include <QApplication>
#include "gamescene.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建主窗口
QWidget window;
window.setFixedSize(400, 400);
// 创建游戏场景
GameScene gameScene(&window);
gameScene.setGeometry(0, 0, 400, 400);
gameScene.setFocus();
// 显示窗口
window.show();
return a.exec();
}
```
这是一个简单的贪吃蛇游戏示例,你可以根据自己的理解和需求对代码进行修改和扩展。
相关推荐
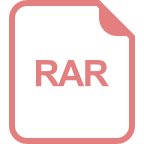
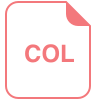













