写一段c语言程序 找共同 题目描述 给你两个序列,长度相等 请找出两个序列中共同出现的元素 由于有多种输出方式,这里我们要求按照所有共同出现的元素从小到大顺序输出,如果重复多次,也只用输出一次,如果一个共同出现的数都没有,输出0即可。 题目保证序列中的元素一定是正整数 输入 第一行一个正整数t,表示有t组数据 每一组第一行一个正整数n,表示序列的长度,序列长度不超过100 每一组第二行和第三行分别为两个序列的元素,序列中每个元素都不超过100, 输出 每一组数据输出两个序列中共同出现的元素
时间: 2024-02-11 13:09:19 浏览: 24
好,这里提供一份使用哈希表的 C 语言实现示例:
```
#include <stdio.h>
#include <stdbool.h>
#define MAXN 100
// 哈希表节点定义
struct HashNode {
int key;
bool value;
struct HashNode* next;
};
// 哈希表定义
struct HashTable {
struct HashNode* buckets[101];
};
// 创建哈希表节点
struct HashNode* create_hash_node(int key, bool value) {
struct HashNode* node = (struct HashNode*)malloc(sizeof(struct HashNode));
node->key = key;
node->value = value;
node->next = NULL;
return node;
}
// 向哈希表中插入一个键值对
void insert_into_hash_table(struct HashTable* ht, int key, bool value) {
int index = key % 101;
struct HashNode* node = ht->buckets[index];
while (node != NULL) {
if (node->key == key) {
node->value = value;
return;
}
node = node->next;
}
struct HashNode* new_node = create_hash_node(key, value);
new_node->next = ht->buckets[index];
ht->buckets[index] = new_node;
}
// 从哈希表中查找一个键的值
bool find_in_hash_table(struct HashTable* ht, int key) {
int index = key % 101;
struct HashNode* node = ht->buckets[index];
while (node != NULL) {
if (node->key == key) {
return node->value;
}
node = node->next;
}
return false;
}
// 销毁哈希表
void destroy_hash_table(struct HashTable* ht) {
for (int i = 0; i < 101; i++) {
struct HashNode* node = ht->buckets[i];
while (node != NULL) {
struct HashNode* next = node->next;
free(node);
node = next;
}
}
}
int main() {
int t;
scanf("%d", &t);
while (t--) {
int n, a[MAXN], b[MAXN];
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
for (int i = 0; i < n; i++) {
scanf("%d", &b[i]);
}
struct HashTable* ht = (struct HashTable*)malloc(sizeof(struct HashTable)); // 创建哈希表
memset(ht->buckets, 0, sizeof(ht->buckets)); // 初始化哈希表
for (int i = 0; i < n; i++) {
insert_into_hash_table(ht, a[i], true); // 将第一个序列中的所有元素插入哈希表中
}
int found = 0; // 标记是否找到了共同出现的元素
for (int i = 0; i < n; i++) {
if (find_in_hash_table(ht, b[i])) { // 如果当前元素在第一个序列中出现过
if (!found || b[i] != found) { // 如果还没有找到共同出现的元素,或者找到了一个不同的元素
printf("%d ", b[i]); // 输出这个元素
found = b[i]; // 更新 found
}
}
}
if (!found) { // 如果没有找到共同出现的元素
printf("0");
}
printf("\n");
destroy_hash_table(ht); // 销毁哈希表
}
return 0;
}
```
这段代码中使用了一个哈希表来记录每个数是否出现过,从而实现了查找共同出现的元素。具体实现时,先将第一个序列中的所有元素插入到哈希表中,然后遍历第二个序列中的所有元素,对于每个元素,如果它在哈希表中出现过,则说明它是共同出现的元素之一,将其输出即可。最后如果没有找到共同出现的元素,则输出 0。
相关推荐
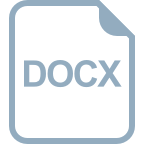
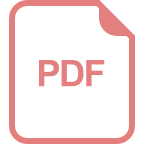














