使用paddle写一段分类神经网络,数据输入输出为:输入为20*25的二维矩阵,输出为14类的分类
时间: 2023-06-17 09:08:05 浏览: 40
好的,以下是一段使用PaddlePaddle实现的分类神经网络代码,数据输入为20*25的二维矩阵,输出为14类的分类。
```python
import paddle
import paddle.fluid as fluid
# 定义输入层
input = fluid.layers.data(name='input', shape=[20, 25], dtype='float32')
# 定义第一层全连接层,输出维度为256
fc1 = fluid.layers.fc(input=input, size=256, act='relu')
# 定义第二层全连接层,输出维度为128
fc2 = fluid.layers.fc(input=fc1, size=128, act='relu')
# 定义输出层全连接层,输出维度为14
output = fluid.layers.fc(input=fc2, size=14, act='softmax')
# 定义损失函数
label = fluid.layers.data(name='label', shape=[1], dtype='int64')
cost = fluid.layers.cross_entropy(input=output, label=label)
avg_cost = fluid.layers.mean(cost)
# 定义优化函数
optimizer = fluid.optimizer.AdamOptimizer(learning_rate=0.001)
optimizer.minimize(avg_cost)
# 定义预测程序
test_program = fluid.default_main_program().clone(for_test=True)
# 定义训练程序
batch_size = 32
epochs = 10
train_reader = paddle.batch(paddle.reader.shuffle(paddle.dataset.uci_housing.train(), buf_size=500), batch_size=batch_size)
place = fluid.CPUPlace()
exe = fluid.Executor(place)
exe.run(fluid.default_startup_program())
# 开始训练
for epoch_id in range(epochs):
for batch_id, data in enumerate(train_reader()):
x_data = np.array([item[0] for item in data], dtype='float32').reshape(-1, 20, 25)
y_data = np.array([item[1] for item in data], dtype='int64').reshape(-1, 1)
outs = exe.run(feed={'input': x_data, 'label': y_data},
fetch_list=[avg_cost])
avg_loss = np.mean(outs[0])
if batch_id % 20 == 0:
print("Epoch {} Batch {} Loss {}".format(epoch_id, batch_id, avg_loss))
# 保存模型
fluid.io.save_inference_model(dirname='./model', feeded_var_names=['input'], target_vars=[output], executor=exe)
```
解释一下代码:
首先,我们定义了一个输入层,接着定义了两个全连接层,最后是输出层,输出层使用了softmax函数进行分类,其中每一层的激活函数都是ReLU函数。
接下来,我们定义了损失函数和优化函数,损失函数使用的是交叉熵,优化函数使用Adam优化器。
然后,我们定义了预测程序和训练程序。训练时,我们使用了UCI Housing数据集,每个batch的大小为32,训练10个epochs。
最后,我们用PaddlePaddle的API保存了训练好的模型。
相关推荐
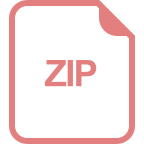
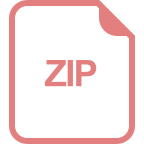
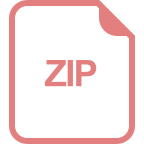














