python实现双种群遗传算法详细代码
时间: 2024-11-05 17:11:53 浏览: 2
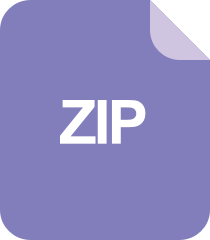
双种群遗传算法.zip

Python实现双种群遗传算法(Differential Evolution,DE)通常涉及到以下几个步骤:
1. **初始化种群**:创建两个大小相同的种群,每个种群包含一系列随机生成的解决方案(个体),称为“解向量”。
```python
import numpy as np
def initialize_population(pop_size, problem_dim):
pop1 = np.random.uniform(lower_bound, upper_bound, (pop_size, problem_dim))
pop2 = np.random.uniform(lower_bound, upper_bound, (pop_size, problem_dim))
return pop1, pop2
```
2. **适应度计算**:根据目标函数对每个解向量评估其适应度。
```python
def fitness_function(solution):
# 根据具体优化问题的函数定义
return objective_function(solution)
```
3. **变异操作**:从两个种群中选择个体,并进行变异生成新的解向量。
```python
def mutation(pop1, pop2, F):
for i in range(pop1.shape[0]):
a, b, c = select_individuals(pop1, pop2)
mutant = a + F * (b - c)
if check_boundary(mutant): # 遵守边界条件
pop1[i] = mutant
```
4. **交叉操作**:结合两个种群进行重组。
```python
def crossover(pop1, pop2, CR):
offspring1, offspring2 = [], []
for _ in range(pop1.shape[0]):
parent1, parent2 = select_parents(pop1, pop2)
if np.random.rand() < CR:
child = crossover_operator(parent1, parent2)
else:
child = parent1
offspring1.append(child)
offspring2.append(crossover_operator(parent2, parent1)) # 对称交叉
return offspring1, offspring2
```
5. **更新种群**:将新产生的解替换到原种群中的一部分位置。
```python
def update_population(pop1, pop2, offspring1, offspring2):
elite = select_best(pop1, pop2) # 保留精英个体
pop1[elite_index] = offspring1
pop2[elite_index] = offspring2
```
6. **迭代过程**:重复上述步骤直到达到预设的停止条件(如达到最大迭代次数或适应度收敛)。
```python
for iteration in range(max_iterations):
mutated_pop1, mutated_pop2 = mutation(pop1, pop2, F)
crossed_offspring1, crossed_offspring2 = crossover(mutated_pop1, mutated_pop2, CR)
update_population(pop1, pop2, crossed_offspring1, crossed_offspring2)
best_solution = select_best(pop1, pop2)
```
完整的代码示例:
```python
class DE:
def __init__(self, lower_bound, upper_bound, pop_size, max_iterations, F, CR):
self.lower_bound, self.upper_bound = lower_bound, upper_bound
self.pop_size = pop_size
self.max_iterations = max_iterations
self.F = F
self.CR = CR
self.pop1, self.pop2 = initialize_population(self.pop_size, problem_dim)
def run(self):
# ... 执行遗传算法的迭代过程 ...
if __name__ == "__main__":
de_algorithm = DE(...args...)
best_solution = de_algorithm.run()
```
阅读全文
相关推荐
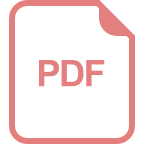


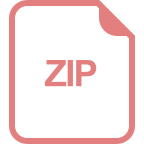
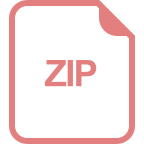
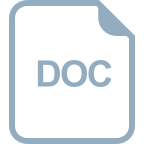
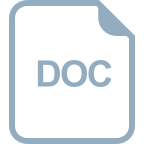









