python实现LBP图像特征提取
时间: 2023-07-11 20:04:52 浏览: 59
LBP(Local Binary Patterns)是一种用于图像纹理分析的特征描述符,它可以用于人脸识别、纹理分类等领域。下面是Python实现LBP图像特征提取的示例代码:
```python
import cv2
import numpy as np
# LBP算法实现
def LBP(img):
h, w = img.shape[:2]
result = np.zeros((h-2, w-2), dtype=np.uint8)
for i in range(1, h-1):
for j in range(1, w-1):
center = img[i][j]
code = []
code.append(int(img[i-1][j-1] >= center))
code.append(int(img[i-1][j] >= center))
code.append(int(img[i-1][j+1] >= center))
code.append(int(img[i][j+1] >= center))
code.append(int(img[i+1][j+1] >= center))
code.append(int(img[i+1][j] >= center))
code.append(int(img[i+1][j-1] >= center))
code.append(int(img[i][j-1] >= center))
code = np.array(code, dtype=np.uint8)
result[i-1][j-1] = np.packbits(code)
return result
# 读取图像
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
# 计算LBP特征图像
lbp = LBP(img)
# 显示原始图像和LBP特征图像
cv2.imshow('image', img)
cv2.imshow('lbp', lbp)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,首先定义了LBP算法实现的函数LBP,该函数接收一张灰度图像作为输入,并返回计算得到的LBP特征图像。在函数内部,使用两个嵌套循环遍历图像中的每个像素点,对于每个像素点,计算它周围8个像素点的LBP编码,最后将8个二进制值拼接成一个8位的二进制数,并将其转化为一个字节存储在结果图像中。
接下来,读取输入图像并调用LBP函数计算得到LBP特征图像,最后使用OpenCV库显示原始图像和LBP特征图像。
相关推荐
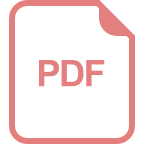
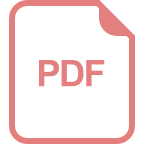
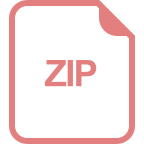
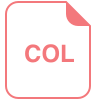
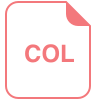
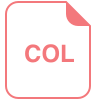
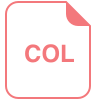
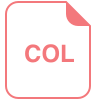









