LBP图像特征提取分类python
时间: 2023-10-08 14:08:04 浏览: 118
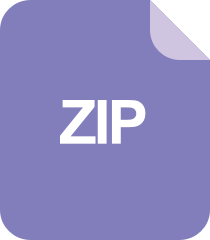
一组使用稀疏编码算法 进行特征提取和图像分类 的 Python工具_python_代码_下载

LBP(Local Binary Pattern)是一种用于图像纹理分析的局部特征描述符。LBP算法可以用于图像分类、人脸识别等领域。下面是一个简单的LBP图像特征提取和分类的python代码示例:
```python
import numpy as np
import cv2
from sklearn.svm import LinearSVC
from sklearn.metrics import accuracy_score
# 计算LBP特征
def calc_lbp_feature(img):
rows, cols = img.shape
lbp_feature = np.zeros((rows-2, cols-2))
for i in range(1, rows-1):
for j in range(1, cols-1):
center = img[i, j]
code = 0
code |= (img[i-1, j-1] > center) << 7
code |= (img[i-1, j] > center) << 6
code |= (img[i-1, j+1] > center) << 5
code |= (img[i, j+1] > center) << 4
code |= (img[i+1, j+1] > center) << 3
code |= (img[i+1, j] > center) << 2
code |= (img[i+1, j-1] > center) << 1
code |= (img[i, j-1] > center) << 0
lbp_feature[i-1, j-1] = code
return lbp_feature.flatten()
# 加载数据集
def load_dataset():
images = []
labels = []
for i in range(1, 41): # 40个人
for j in range(1, 11): # 每个人10张图片
img = cv2.imread(f"att_faces/s{i}/{j}.pgm", cv2.IMREAD_GRAYSCALE)
images.append(img)
labels.append(i)
return images, labels
# 提取LBP特征并训练分类器
def train_lbp_classifier(images, labels):
data = []
for img in images:
feature = calc_lbp_feature(img)
data.append(feature)
clf = LinearSVC()
clf.fit(data, labels)
return clf
# 测试分类器
def test_lbp_classifier(clf, images, labels):
pred_labels = []
for img in images:
feature = calc_lbp_feature(img)
pred_label = clf.predict([feature])[0]
pred_labels.append(pred_label)
acc = accuracy_score(labels, pred_labels)
print(f"Accuracy: {acc}")
if __name__ == "__main__":
images, labels = load_dataset()
clf = train_lbp_classifier(images, labels)
test_lbp_classifier(clf, images, labels)
```
在这个示例中,我们使用了AT&T人脸数据集中的图像进行训练和测试。在训练过程中,我们提取了每个图像的LBP特征,并使用LinearSVC(线性支持向量机)训练了一个分类器。在测试过程中,我们使用相同的LBP特征来对测试图像进行分类,并计算了分类器的准确率。
阅读全文
相关推荐



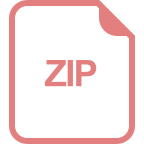
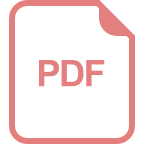
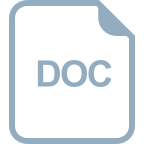









