Java在Shape类基础上设计圆形,矩形子类,要求根据实际形状重写getArea()方法,并设计一个TestShape类,通过调用countArea方法把两个对象面积累加到area中,最后输出area
时间: 2024-10-25 08:09:45 浏览: 51
在Java中,我们可以继续在`Shape`类的基础上扩展出`Circle`和`Rectangle`子类,并在`TestShape`类中使用这两个子类的对象来计算它们的面积总和。这是一个示例:
首先,我们有`Shape`抽象类:
```java
abstract class Shape {
abstract double getArea();
void printArea() {
System.out.println("Area of the shape is: " + getArea());
}
}
```
然后,是`Circle`和`Rectangle`子类:
```java
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double getArea() {
return Math.PI * radius * radius;
}
}
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
double getArea() {
return width * height;
}
}
```
接着是`TestShape`类,它有一个`countArea`方法用于累加两个形状的面积:
```java
public class TestShape {
private double area = 0;
public void countArea(Shape circle, Shape rectangle) {
area += circle.getArea();
area += rectangle.getArea();
}
public void printTotalArea() {
System.out.println("Total area of both shapes is: " + area);
}
public static void main(String[] args) {
TestShape test = new TestShape();
Circle circle = new Circle(5);
Rectangle rectangle = new Rectangle(4, 6);
test.countArea(circle, rectangle);
test.printTotalArea();
}
}
```
当你运行`main`方法时,会先创建一个`Circle`和一个`Rectangle`对象,然后将它们的面积加入`TestShape`类的`area`变量中,并最终打印出总面积。
阅读全文
相关推荐
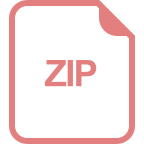
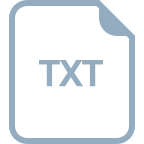
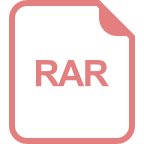















