windows下如何写消息队列C++
时间: 2024-04-21 21:22:47 浏览: 8
在Windows下,可以使用Windows API中的命名管道(Named Pipes)来实现进程间的消息队列。以下是一个简单的示例代码,演示了如何在C++中创建命名管道、向管道写入消息以及从管道读取消息。
```cpp
#include <iostream>
#include <windows.h>
#define PIPE_NAME "\\\\.\\pipe\\my_named_pipe" // 命名管道的名称
#define BUFFER_SIZE 1024
int main() {
// 创建命名管道
HANDLE pipe = CreateNamedPipe(
PIPE_NAME, // 管道名称
PIPE_ACCESS_DUPLEX, // 可读写访问权限
PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE | PIPE_WAIT, // 消息类型管道,消息模式读取,阻塞模式
PIPE_UNLIMITED_INSTANCES, // 允许多个实例
BUFFER_SIZE, // 输入缓冲区大小
BUFFER_SIZE, // 输出缓冲区大小
0, // 默认超时时间
NULL // 默认安全属性
);
if (pipe == INVALID_HANDLE_VALUE) {
std::cerr << "Failed to create named pipe." << std::endl;
return 1;
}
// 连接到命名管道
if (!ConnectNamedPipe(pipe, NULL)) {
std::cerr << "Failed to connect to named pipe." << std::endl;
CloseHandle(pipe);
return 1;
}
// 向管道写入消息
std::string message = "Hello, another process!";
DWORD bytesWritten;
if (!WriteFile(pipe, message.c_str(), message.size() + 1, &bytesWritten, NULL)) {
std::cerr << "Failed to write to named pipe." << std::endl;
CloseHandle(pipe);
return 1;
}
// 从管道读取消息
char buffer[BUFFER_SIZE];
DWORD bytesRead;
if (!ReadFile(pipe, buffer, BUFFER_SIZE, &bytesRead, NULL)) {
std::cerr << "Failed to read from named pipe." << std::endl;
CloseHandle(pipe);
return 1;
}
std::cout << "Received message: " << buffer << std::endl;
// 关闭命名管道
CloseHandle(pipe);
return 0;
}
```
在上述示例中,我们使用`CreateNamedPipe`函数创建了一个命名管道,并指定了管道的名称、访问权限、消息类型、缓冲区大小等参数。然后,我们使用`ConnectNamedPipe`函数来连接到命名管道。
接下来,我们使用`WriteFile`函数向管道写入消息,以及使用`ReadFile`函数从管道读取消息。最后,我们使用`CloseHandle`函数关闭命名管道。
请注意,这只是一个简单的示例,实际使用中可能还需要处理错误、同步、超时等方面的问题。另外,如果需要在多个进程之间进行通信,可以通过创建多个命名管道来实现。
相关推荐
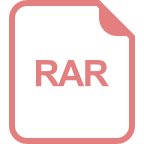
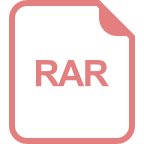














