设计一个程序,首先确在屏幕的中央设置圆心,生成一个半径为200的圆,之后以相同的圆心生成一个半径为200的直角扇形。此时,在圆内随机生成一个黑点,设置一个翻转按钮,按下此按钮,将扇形的中心移动到黑点处,并将此扇形全部变为红色,并且在控制台输出黑点的坐标和与圆心的角度
时间: 2024-05-31 16:07:19 浏览: 16
以下是Python的实现代码:
import pygame
import math
import random
# 初始化pygame
pygame.init()
# 设置屏幕大小和标题
size = (800, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("圆形和扇形")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 设置字体
font = pygame.font.Font(None, 36)
# 定义圆心
center = (400, 300)
# 定义圆的半径
radius = 200
# 生成圆的surface
circle_surface = pygame.Surface((size[0], size[1]), pygame.SRCALPHA)
pygame.draw.circle(circle_surface, BLACK, center, radius, 1)
# 定义扇形的角度
angle = 45
# 生成扇形的surface
sector_surface = pygame.Surface((size[0], size[1]), pygame.SRCALPHA)
pygame.draw.arc(sector_surface, BLACK, (center[0]-radius, center[1]-radius, radius*2, radius*2), math.radians(-angle/2), math.radians(angle/2), 1)
pygame.draw.line(sector_surface, BLACK, center, (center[0], center[1]-radius), 1)
# 随机生成黑点
black_point = (random.randint(center[0]-radius, center[0]+radius), random.randint(center[1]-radius, center[1]+radius))
pygame.draw.circle(sector_surface, BLACK, black_point, 5)
# 定义是否翻转的标志
flipped = False
# 游戏循环
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
flipped = not flipped
# 计算扇形中心点
if flipped:
sector_center = black_point
else:
sector_center = center
# 计算黑点与圆心的角度
dx = black_point[0] - center[0]
dy = black_point[1] - center[1]
angle = math.degrees(math.atan2(dy, dx))
if angle < 0:
angle += 360
# 输出信息到控制台
print("黑点坐标:", black_point)
print("与圆心的角度:", angle)
# 清屏
screen.fill(WHITE)
# 绘制圆和扇形
if flipped:
sector_color = RED
else:
sector_color = BLACK
circle_surface.blit(sector_surface, (0, 0), special_flags=pygame.BLEND_RGBA_MIN)
pygame.draw.circle(circle_surface, sector_color, sector_center, radius, 1)
screen.blit(circle_surface, (0, 0))
# 绘制翻转按钮
if flipped:
button_text = "翻回来"
else:
button_text = "翻转"
button_surface = font.render(button_text, True, BLACK)
button_rect = button_surface.get_rect(center=(size[0]-100, 50))
pygame.draw.rect(screen, WHITE, button_rect)
pygame.draw.rect(screen, BLACK, button_rect, 1)
screen.blit(button_surface, button_rect)
# 更新屏幕
pygame.display.flip()
# 退出pygame
pygame.quit()
相关推荐
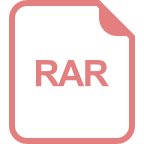
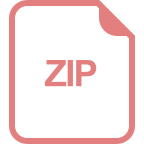














