unity TryGetValue
时间: 2024-10-17 19:15:37 浏览: 21
`Unity.TryGetValue()`是一个方法,通常在.NET框架特别是C#语言中的Dictionary、Hashtable等集合类型的实现中找到。这个方法用于尝试从给定的键值对存储结构中获取值,而不会引发异常,如果指定的键不存在,它会返回默认值而不是抛出KeyNotFoundException。
例如,在C#的Dictionary中,你可以这样做:
```csharp
Dictionary<string, int> myDict = new Dictionary<string, int>();
int value;
if (myDict.TryGetValue("key", out value))
{
// 如果键存在,value会被赋值并返回true
Console.WriteLine($"Found value: {value}");
}
else
{
// 键不存在,value将保持默认值,且返回false
Console.WriteLine("Key not found");
}
```
在这里,`out`关键字用于声明一个变量,并表示该方法将把结果赋值给它,如果键存在则赋值成功,否则变量保持未初始化的状态。
相关问题
unity Dictionary使用
在Unity中,虽然官方没有提供内置的Dictionary数据结构,但我们可以利用C#语言自带的`System.Collections.Generic.Dictionary<TKey, TValue>`来存储和操作键值对。以下是如何在Unity脚本中使用它的一般步骤:
1. **创建Dictionary实例**:
```csharp
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
```
这里创建了一个字符串到整数类型的字典。
2. **添加元素**:
```csharp
myDictionary.Add("Key1", 5);
```
或者你可以直接初始化:
```csharp
myDictionary = new Dictionary<string, int> { { "Key1", 5 }, { "Key2", 10 } };
```
3. **访问元素**:
```csharp
int value;
if (myDictionary.TryGetValue("Key1", out value))
{
Debug.Log($"Value of Key1 is: {value}");
}
```
`TryGetValue`用于安全地获取键对应的值,如果没有则返回默认值。
4. **删除元素**:
```csharp
myDictionary.Remove("Key1");
```
5. **遍历Dictionary**:
```csharp
foreach (KeyValuePair<string, int> entry in myDictionary)
{
Debug.Log($"Key: {entry.Key}, Value: {entry.Value}");
}
```
6. **检查是否存在键**:
```csharp
bool containsKey = myDictionary.ContainsKey("Key1");
```
Unity 字典查找
在Unity引擎中,字典(Dictionary)是一种用于存储键值对的数据结构,它允许通过唯一的键(Key)快速查找对应的值(Value)。在C#中,Unity内置了System.Collections.Generic命名空间下的Dictionary类,比如Dictionary<TKey, TValue>,TKey是键的类型,TValue是值的类型。
例如,你可以创建一个字符串到颜色的字典:
```csharp
Dictionary<string, Color> colorDictionary = new Dictionary<string, Color>();
colorDictionary.Add("Red", Color.red);
colorDictionary.Add("Green", Color.green);
// 查找特定颜色
Color lookupColor;
if (colorDictionary.TryGetValue("Blue", out lookupColor))
{
// 如果有找到,lookupColor就是蓝色
}
else
{
// 如果没有找到,lookupColor将是默认值或抛出异常
}
```
查找时,TryGetValue方法可以帮助避免null值检查,并返回是否成功查找到了值。如果键不存在,它会提供一个默认值(如上述示例中的`out lookupColor`)或者抛出异常。
阅读全文
相关推荐
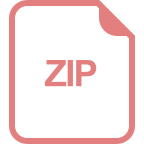












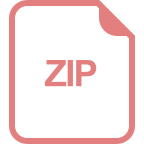
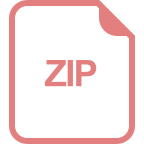
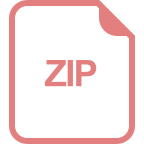