tcn代码python
时间: 2023-11-15 20:02:41 浏览: 60
TCN是Temporal Convolutional Network的缩写,是一种用于处理时间序列数据的神经网络模型。它利用卷积神经网络(CNN)的思想来构建时间卷积层,通过捕捉时间上的局部依赖关系来提取序列中的特征。
在Python中,我们可以使用TensorFlow或PyTorch等深度学习库来实现TCN模型。以下是一个基本的TCN代码示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv1D, Dense
class TCN(tf.keras.Model):
def __init__(self, input_dim, output_dim, num_channels, kernel_size):
super(TCN, self).__init__()
self.conv_layers = []
for i, num_channels in enumerate(num_channels):
self.conv_layers.append(Conv1D(num_channels, kernel_size, padding='same', activation='relu'))
self.fc = Dense(output_dim, activation='softmax')
def call(self, x):
for conv in self.conv_layers:
x = conv(x)
x = tf.reduce_mean(x, axis=1) # Global average pooling
x = self.fc(x)
return x
# 创建TCN模型实例
input_dim = 10 # 输入维度
output_dim = 5 # 输出维度
num_channels = [64, 32, 16] # 每个卷积层的通道数
kernel_size = 3 # 卷积核大小
model = TCN(input_dim, output_dim, num_channels, kernel_size)
# 编译模型,指定损失函数和优化器
model.compile(loss='categorical_crossentropy', optimizer='adam')
# 训练模型
model.fit(train_data, train_labels, epochs=10, batch_size=32)
# 使用模型进行预测
predictions = model.predict(test_data)
```
在这个代码示例中,我们首先定义了一个TCN类作为模型,在`__init__`方法中使用Conv1D层来构建卷积层。然后在`call`方法中,我们逐层进行卷积操作,并进行全局平均池化操作。最后使用全连接层将提取的特征映射到输出维度,并返回预测结果。
该模型需要指定输入维度、输出维度、每个卷积层的通道数以及卷积核大小。然后通过`model.compile`方法指定损失函数和优化器,使用`model.fit`方法进行模型训练。最后使用`model.predict`方法对测试数据进行预测。
相关推荐
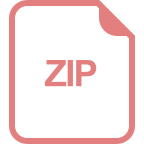
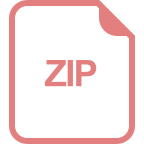














