yolov5实时检测屏幕python代码
时间: 2023-10-10 21:08:59 浏览: 57
以下是使用 YOLOv5 进行实时屏幕检测的 Python 代码:
```python
import cv2
import numpy as np
import time
import pyautogui
# 加载 YOLOv5 模型
model = cv2.dnn.readNet("yolov5.weights", "yolov5.cfg")
# 获取屏幕分辨率
screen_size = pyautogui.size()
width, height = screen_size.width, screen_size.height
# 设置 YOLOv5 输入图像大小
model.setInputSize((640, 640))
# 定义 COCO 数据集的类别标签
class_names = []
with open("coco.names", "r") as f:
class_names = [cname.strip() for cname in f.readlines()]
# 定义 YOLOv5 模型的输出层名称
layer_names = model.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in model.getUnconnectedOutLayers()]
# 打开屏幕捕获
cap = cv2.VideoCapture(0)
while True:
# 读取屏幕图像
ret, frame = cap.read()
# 将屏幕图像转换为 YOLOv5 可以处理的格式
blob = cv2.dnn.blobFromImage(frame, 1 / 255.0, (640, 640), swapRB=True, crop=False)
# 通过 YOLOv5 模型进行目标检测
model.setInput(blob)
start = time.time()
outputs = model.forward(output_layers)
end = time.time()
# 处理模型的输出结果
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制 (NMS)
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(boxes), 3))
if len(indexes) > 0:
for i in indexes.flatten():
x, y, w, h = boxes[i]
label = str(class_names[class_ids[i]])
confidence = str(round(confidences[i], 2))
color = colors[i]
cv2.rectangle(frame, (x, y), (x + w, y + h), color, 2)
cv2.putText(frame, label + " " + confidence, (x, y + 20), font, 2, color, 2)
# 显示检测结果
cv2.imshow("Screen Capture", frame)
# 等待退出
key = cv2.waitKey(1)
if key == 27:
break
# 关闭屏幕捕获和窗口
cap.release()
cv2.destroyAllWindows()
```
请注意,此代码仅在 Windows 操作系统上进行测试。在其他操作系统上可能需要进行修改才能正常工作。此外,此代码未进行优化,可能会导致检测速度较慢。如果需要更快的检测速度,可以尝试使用 YOLOv5 的 GPU 版本或使用其他目标检测算法。
相关推荐
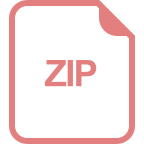
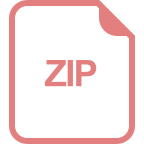
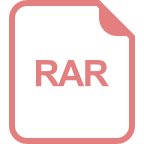














