用python写一个简单俄罗斯方块游戏
时间: 2023-12-31 21:07:11 浏览: 72
好的,下面是一个简单的俄罗斯方块游戏的代码,使用了pygame库来实现游戏界面和交互。
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏界面大小
screen_width = 480
screen_height = 640
# 方块大小和边距
block_size = 20
block_margin = 5
# 游戏界面初始化
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("俄罗斯方块")
# 颜色定义
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 方块种类定义
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[2, 2], [2, 2]],
[[0, 3, 3], [3, 3, 0]],
[[4, 4, 0], [0, 4, 4]],
[[5, 5, 5, 5]],
[[6, 6, 6], [0, 0, 6]],
[[7, 7, 7], [7, 0, 0]],
]
# 方块颜色定义
colors = [
blue,
green,
red,
white,
blue,
green,
red,
]
# 随机生成一个方块
def new_block():
shape = random.choice(shapes)
color = random.choice(colors)
block = {
"shape": shape,
"color": color,
"row": 0,
"col": screen_width // 2 // (block_size + block_margin),
}
return block
# 方块移动
def move_block(block, row, col):
block["row"] += row
block["col"] += col
# 方块旋转
def rotate_block(block):
old_shape = block["shape"]
new_shape = []
for i in range(len(old_shape[0])):
new_row = []
for j in range(len(old_shape)):
new_row.append(old_shape[len(old_shape) - j - 1][i])
new_shape.append(new_row)
block["shape"] = new_shape
# 方块下落
def drop_block(block):
while can_move(block, 1, 0):
move_block(block, 1, 0)
# 判断方块是否可以移动
def can_move(block, row, col):
for i in range(len(block["shape"])):
for j in range(len(block["shape"][0])):
if block["shape"][i][j] != 0:
if block["row"] + i + row >= screen_height // (block_size + block_margin):
return False
if block["col"] + j + col < 0 or block["col"] + j + col >= screen_width // (block_size + block_margin):
return False
if grid[block["row"] + i + row][block["col"] + j + col] != 0:
return False
return True
# 将方块加入到网格中
def merge_block(block):
for i in range(len(block["shape"])):
for j in range(len(block["shape"][0])):
if block["shape"][i][j] != 0:
grid[block["row"] + i][block["col"] + j] = block["color"]
# 消除满行的方块
def remove_full_rows():
num_removed = 0
for i in range(len(grid)):
if all(grid[i]):
del grid[i]
grid.insert(0, [0 for _ in range(screen_width // (block_size + block_margin))])
num_removed += 1
return num_removed
# 绘制方块
def draw_block(block):
for i in range(len(block["shape"])):
for j in range(len(block["shape"][0])):
if block["shape"][i][j] != 0:
x = (block["col"] + j) * (block_size + block_margin)
y = (block["row"] + i) * (block_size + block_margin)
pygame.draw.rect(screen, block["color"], [x, y, block_size, block_size])
# 绘制网格
def draw_grid():
for i in range(len(grid)):
for j in range(len(grid[0])):
x = j * (block_size + block_margin)
y = i * (block_size + block_margin)
pygame.draw.rect(screen, grid[i][j], [x, y, block_size, block_size])
# 游戏结束
def game_over():
font = pygame.font.Font(None, 36)
text = font.render("Game Over", True, red)
screen.blit(text, [screen_width // 2 - text.get_width() // 2, screen_height // 2 - text.get_height() // 2])
pygame.display.flip()
pygame.time.wait(3000)
pygame.quit()
exit()
# 初始化网格
grid = [[0 for _ in range(screen_width // (block_size + block_margin))] for _ in range(screen_height // (block_size + block_margin))]
# 初始化游戏变量
clock = pygame.time.Clock()
fall_time = 0
fall_speed = 0.5
score = 0
# 随机生成一个方块
block = new_block()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and can_move(block, 0, -1):
move_block(block, 0, -1)
elif event.key == pygame.K_RIGHT and can_move(block, 0, 1):
move_block(block, 0, 1)
elif event.key == pygame.K_DOWN and can_move(block, 1, 0):
move_block(block, 1, 0)
elif event.key == pygame.K_UP:
rotate_block(block)
elif event.key == pygame.K_SPACE:
drop_block(block)
# 绘制界面
screen.fill(black)
draw_grid()
draw_block(block)
pygame.display.flip()
# 方块下落
fall_time += clock.get_rawtime()
if fall_time / 1000 > fall_speed:
fall_time = 0
if can_move(block, 1, 0):
move_block(block, 1, 0)
else:
merge_block(block)
num_removed = remove_full_rows()
score += num_removed * 10
if score >= 100:
fall_speed = 0.4
if score >= 200:
fall_speed = 0.3
if score >= 300:
fall_speed = 0.2
if not can_move(new_block(), 0, 0):
game_over()
block = new_block()
# 控制帧率
clock.tick(60)
```
运行代码会打开一个窗口,按左右键移动方块,按上键旋转方块,按下键加速方块下落,按空格键直接将方块下落到底部。游戏规则和普通的俄罗斯方块游戏相同,消除满行的方块可以得分,当网格顶部出现方块时游戏结束。
阅读全文
相关推荐
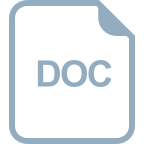
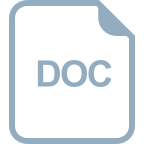
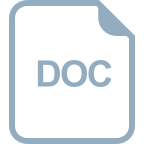










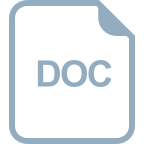
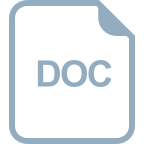
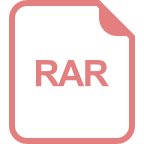
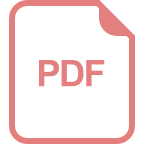
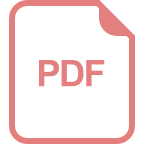