We have HW cards arranged in a matrix with H rows and W columns. For each i=1,…,N, the card at the A i -th row from the top and B i -th column from the left has a number i written on it. The other HW−N cards have nothing written on them. We will repeat the following two kinds of operations on these cards as long as we can. If there is a row without a numbered card, remove all the cards in that row and fill the gap by shifting the remaining cards upward. If there is a column without a numbered card, remove all the cards in that column and fill the gap by shifting the remaining cards to the left. Find the position of each numbered card after the end of the process above. It can be proved that these positions are uniquely determined without depending on the order in which the operations are done.c++代码
时间: 2024-02-13 08:05:03 浏览: 107
以下是实现上述问题的 C++ 代码:
```c++
#include<bits/stdc++.h>
using namespace std;
const int maxn=110;
int h,w,n;
int a[maxn][maxn];
int num[maxn*maxn];
int cnt;
int main()
{
cin>>h>>w>>n;
for(int i=1;i<=n;i++)
{
int x,y;
cin>>x>>y;
a[x][y]=i;
}
for(int i=1;i<=h;i++)
for(int j=1;j<=w;j++)
if(a[i][j])
num[a[i][j]]=i;
for(int j=1;j<=w;j++)
{
bool flag=false;
for(int i=1;i<=h;i++)
if(a[i][j])
flag=true;
if(!flag)
{
for(int k=j+1;k<=w;k++)
for(int i=1;i<=h;i++)
if(a[i][k])
{
swap(a[i][k],a[i][j]);
num[a[i][j]]=i;
goto out;
}
}
out:;
}
cnt=0;
for(int i=1;i<=h;i++)
{
bool flag=false;
for(int j=1;j<=w;j++)
if(a[i][j])
flag=true;
if(!flag)
{
cnt++;
for(int k=i+1;k<=h;k++)
for(int j=1;j<=w;j++)
if(a[k][j])
{
swap(a[k][j],a[i][j]);
num[a[i][j]]=i;
goto out2;
}
}
out2:;
}
for(int i=1;i<=n;i++)
cout<<num[i]<<" "<<i<<endl;
return 0;
}
```
首先,我们需要一个二维数组 `a` 用来存储卡牌上的数字,`a[i][j]` 表示第i行第j列的卡牌上的数字,若该卡牌上无数字,则 `a[i][j]=0`。
然后,我们需要一个一维数组 `num` 存储每个数字所在的行数,即 `num[i]` 表示数字i所在的行数。
接着,我们先遍历一遍二维数组 `a`,将 `num` 数组填充好。
之后,我们按照题目要求模拟两种操作。首先,对于没有数字的行,我们需要将该行删除,即将该行以上的所有行下移一行,然后将最上面一行清空。这一步操作可以通过双重循环实现,注意到该操作可能会使得某些数字所在的行数发生改变,因此我们需要在操作时及时更新 `num` 数组。
接下来,对于没有数字的列,我们需要将该列删除,即将该列左边的所有列右移一列,然后将最左边一列清空。同样地,这一步操作也可以通过双重循环实现,注意到该操作可能会使得某些数字所在的行数发生改变,因此我们需要在操作时及时更新 `num` 数组。
最后,我们输出每个数字所在的行数以及数字本身即可。
需要注意的是,为了避免多重循环嵌套导致程序效率低下,我们使用了 `goto` 语句来进行跳转,使得程序在找到符合条件的数后能够直接跳出循环。
阅读全文
相关推荐
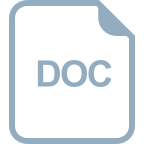
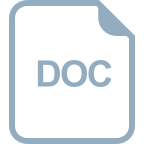
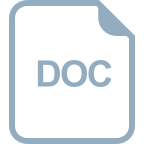
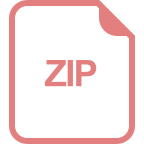
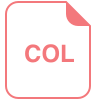
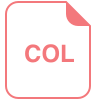
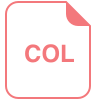
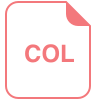
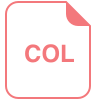









