任意给定5个顶点坐标,利用多边形填充的扫描线算法编写程序,生成一个实心五边形,并且使用OpenGL和C++写出代码
时间: 2024-10-15 15:22:13 浏览: 6
在C++中使用OpenGL绘制实心五边形,通常会结合Bresenham算法来进行多边形填充。首先,你需要了解如何使用OpenGL设置基本的渲染上下文,然后定义一个函数来处理扫描线算法并判断是否在当前线上绘制点。
```cpp
#include <GL/glut.h>
#include <vector>
// Bresenham's algorithm for line drawing
std::vector<int> bresenham(int x0, int y0, int x1, int y1) {
std::vector<int> points;
int dx = abs(x1 - x0);
int dy = abs(y1 - y0);
bool steep = dy > dx;
if (steep) {
swap(x0, y0);
swap(x1, y1);
}
int error = dx - dy;
int y = y0;
for (int x = x0; x <= x1; ++x) {
points.push_back(y);
if (error <= 0) {
y += dy;
error += 2 * dy;
} else {
error -= 2 * dx;
}
}
return points;
}
void draw_polygon(float* vertices, int num_vertices) {
glBegin(GL_POLYGON);
for (int i = 0; i < num_vertices; ++i) {
glVertex2f(vertices[i * 2], vertices[i * 2 + 1]);
// 这里可以用bresenham方法进行填充
// glColor3ub(255, 0, 0); // 红色填充
// glBegin(GL_LINES);
// for (auto point : bresenham(vertices[i * 2], vertices[i * 2 + 1],
// vertices[(i + 1) % num_vertices * 2],
// vertices[(i + 1) % num_vertices * 2 + 1])) {
// glVertex2f(point, vertices[i * 2 + 1]);
// }
// glEnd();
}
glEnd();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutCreateWindow("Filled Pentagon");
glEnable(GL_DEPTH_TEST);
float pentagon[] = { /* 五个顶点的坐标 */ };
draw_polygon(pentagon, 5);
glutDisplayFunc(draw_polygon);
glutMainLoop();
return 0;
}
```
相关推荐
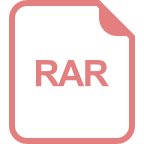
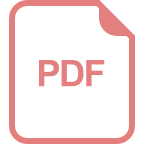



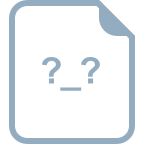
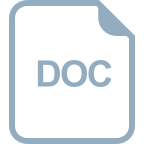




